Mastering Axios Retry Plugin: Handling Failed Requests with Examples
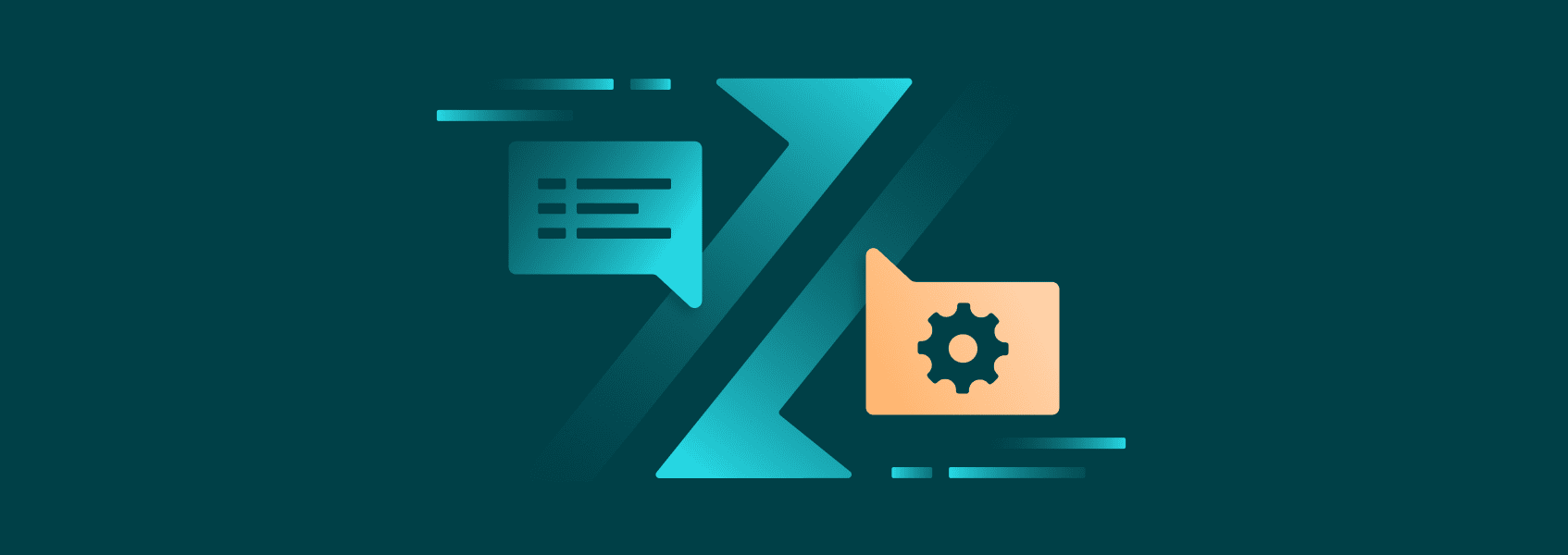
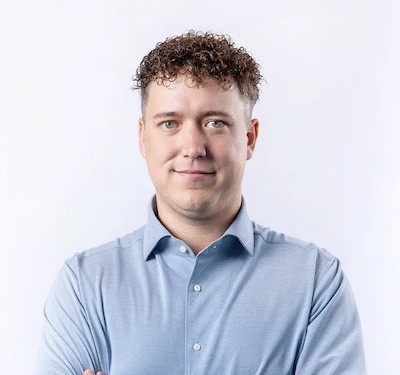
Vilius Dumcius
Axios Retry is a plugin that intercepts failed HTTP requests and retries them based on your configuration. It’s widely used in web development and data scraping applications where failed HTTP requests are quite common.
In general, nearly any software development project that relies on external server communication will have to have some way of retrying requests. Otherwise, the entire project would halt at the first time a network error occurs.
Axios Retry is widely used because it’s intuitive, simple, and supports async function development. You can also code your own custom retry logic, making the plugin extremely flexible.
What Are The Main Features of Axios?
Axios has no in-built failed request management features. If you program an application without the Retry plugin, Axios will simply throw a network exception whenever an error occurs.
You can use “try…catch” to catch a network exception through default JavaScript features (which are available in Axios). That won’t get you far, though, as you’d have to code a way to retry failed HTTP requests from the ground up.
The Axios Retry library is used to bypass the entire process of building a custom retry logic. If you still want to do so, the Axios Retry plugin is immensely useful by shortening the process by a significant margin.
One of the main selling points for Axios Retry is the amount of configuration options for retrying requests. You can set up automatic retries based on HTTP error codes, the amount of retries, a backoff delay, and customize the logic in many other ways.
Most of these features will be incredibly useful whenever any type of automation is happening. In web scraping, for example, the bot has to send requests to thousands if not hundreds of thousands of URLs per day. Network error issues will be frequent, whether it’s due to the client or the server.
Setting up Axios requests would make web scraping much more efficient. Every network error that’s within the configuration settings would be retried while the bot continues its tasks on other URLs. Without Axios requests, the bot would either throw a network exception or would fail to reach some of the URLs.
Practical Axios Retry Example
If you’re starting from scratch, before you can even install Axios Retry in your project, you’ll need Node.JS . Node.JS allows JavaScript to be used anywhere, which is what Axios runs on.
Once that’s installed on your system, open up your IDE and install Axios Retry and Axios itself.
npm install axios axios-retry
If you run into an NPM error, it’s likely that Node.JS hasn’t been installed properly. Otherwise, the packages should be installed correctly.
We can then set up an extremely basic retry system:
const axios = require('axios');
const axiosRetry = require('axios-retry').default;
// Configure axios-retry
axiosRetry(axios, {
retries: 3, // Number of retries
retryCondition: axiosRetry.isRetryableError, // Retry on network errors and 5xx responses
});
axios.get('https://someapi.com/data')
.then(response => console.log(response.data))
.catch(error => console.error('Failed after retries:', error));
Let’s go through the code line by line.
We first import the two packages through the “require” function. NodeJS does have the “import” you may be familiar with, however, it’s not recommended to use it due to it requiring an experimental flag (and for several other reasons).
Regardless of whether you choose “require” or “import”, pick one. Using both is prohibited and most IDEs will automatically disable either one.
We then use the “axiosRetry” function to create an “axios” instance. We do that by including “axios” as one of the arguments for the retry function.
Our second argument is an object (closely related to a dictionary in Python) where we use key:value pairs to set up the configuration. In our case, we create an object with two properties, one of which is “retries” and a numerical value, the other is “retryCondition” and a predicate function that returns true or false.
In simpler terms, imagine we receive a network error while performing actions. Our “axiosRetry” function will intercept the network error, check if it matches known errors or a 5xx response, and if it does, will attempt to establish a connection a total of 3 times.
Finally, “axios.get” is a fairly simple function that’s intended to use Axios to send a GET HTTP request to a URL. If that connection is successful, the “.then()” logs the response data to the console. If an error occurs (including failing on retries), an error message is output instead.
Note that nothing is done for the error message “Request Aborted”. These are requests that users cancel manually. Capturing “Request Aborted” and retrying on them is only likely to cause confusion and difficulties.
Axios Requests Retry with Backoff
We can also include an exponential backoff strategy. Exponential backoff continually increases the delay before sending the next retry. These strategies are useful if a server is temporarily overloaded, for example.
const axios = require('axios');
const axiosRetry = require('axios-retry').default;
// Configure axios-retry with exponential backoff
axiosRetry(axios, {
retries: 3,
retryDelay: axiosRetry.exponentialDelay, // Exponential back-off
retryCondition: axiosRetry.isRetryableError,
});
axios.get('https://someapi.com/data')
.then(response => console.log(response.data))
.catch(error => console.error('Failed after retries with exponential backoff:', error));
We only adjusted our “axiosRetry” object to include a new property “retryDelay”. We’re using the default Axios requests retry backoff delay, which is 2n * 100 ms, where “n” is the number of retries initiated.
If you need a custom backoff strategy, the value “axiosRetry.exponentialDelay” can be modified to refer to a custom function:
const axios = require('axios');
const axiosRetry = require('axios-retry').default;
// Custom exponential backoff function
function customExponentialBackoff(retryNumber) {
// Start with 200ms delay, double each retry
return 200 * Math.pow(2, retryNumber);
}
// Configure axios-retry
axiosRetry(axios, {
retries: 3,
retryDelay: customExponentialBackoff, // Use custom backoff function
retryCondition: axiosRetry.isRetryableError,
});
// Make a GET request
axios.get('https://someapi.com/data')
.then(response => console.log(response.data))
.catch(error => console.error('Failed after retries with custom exponential backoff:', error));
Customizing Retry Conditions
Axios Retry also provides numerous ways to modify the retry conditions. First, we can modify the logic to only retry on specific error codes:
const axios = require('axios');
const axiosRetry = require('axios-retry').default;
// Custom retry condition
axiosRetry(axios, {
retries: 4,
retryCondition: (error) => {
return error.response ? error.response.status === 503 : false;
},
});
axios.get('https://someapi.com/data')
.then(response => console.log(response.data))
.catch(error => console.error('Failed after retries on 503:', error));
We modify the “retryCondition” key this time by taking the “(error)” value (which is also an error object) and build a conditional statement with “?” and “:”. Our conditional states that if an error response is received, then the status code is checked and if it is equal to 503 (Temporarily Unavailable), the conditional returns “true”. Otherwise, “false” is returned.
We can also modify the logic to only retry on specific HTTP methods:
const axios = require('axios');
const axiosRetry = require('axios-retry').default;
// Configure axios-retry to apply only to POST requests
axiosRetry(axios, {
retries: 3,
shouldRetry: (config) => {
return config.method === 'post';
}
});
axios.post('https://someapi.com/submit', { data: 'example' })
.then(response => console.log('Data submitted:', response.data))
.catch(error => console.error('Failed after retries for POST:', error));
Now, if our application is sending various requests, only failed POST requests will be retried. These are generally useful as a redundancy for important modification tasks on a server.
Conclusion
Axios Retry is a great plugin if you need a simple, intuitive, and high performance package to retry failed requests on. It also receives major community support and has a lot of discussions online, so finding working code examples or asking questions is a lot easier than for some of the more obscure libraries.
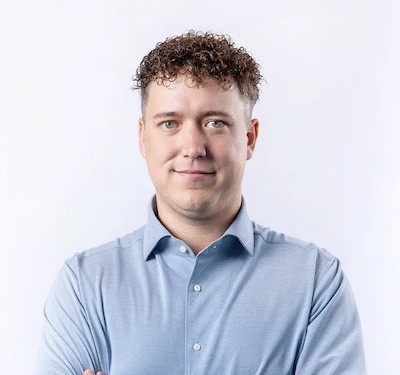
Author
Vilius Dumcius
Product Owner
With six years of programming experience, Vilius specializes in full-stack web development with PHP (Laravel), MySQL, Docker, Vue.js, and Typescript. Managing a skilled team at IPRoyal for years, he excels in overseeing diverse web projects and custom solutions. Vilius plays a critical role in managing proxy-related tasks for the company, serving as the lead programmer involved in every aspect of the business. Outside of his professional duties, Vilius channels his passion for personal and professional growth, balancing his tech expertise with a commitment to continuous improvement.
Learn More About Vilius Dumcius