How to Use the Google Image Search API: A Complete Guide
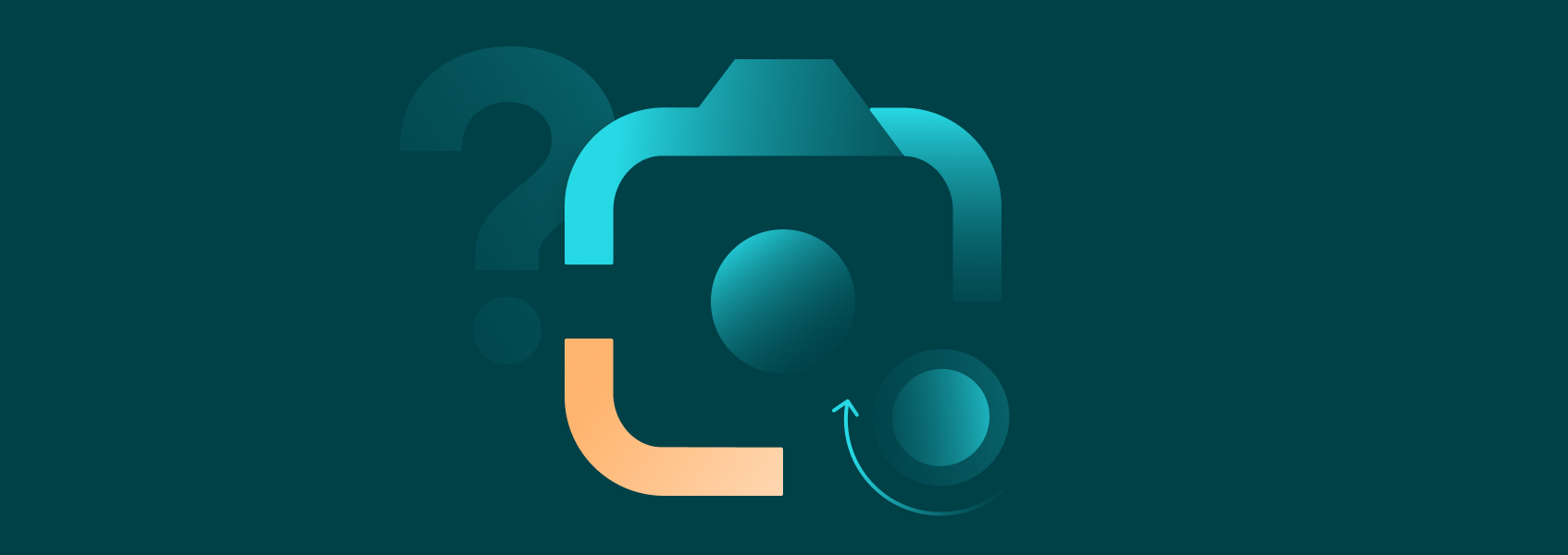
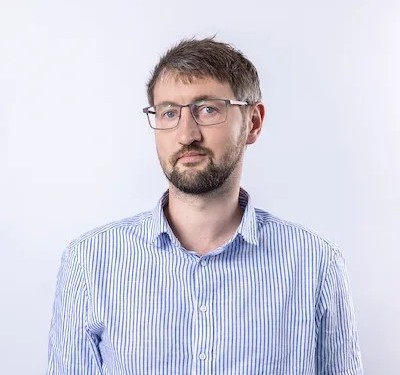
Nerijus Kriaučiūnas
In This Article
Google Image Search API was a dedicated application programming interface that allowed users to search and potentially download images through code. Unfortunately, it has since been deprecated as no mention, documentation, or access exists.
There’s a workaround, though, which is using the Google Custom Search API with a programmable custom search engine (CSE). Using the Search API properly will allow you to do everything Google Image Search API would have allowed you to. In fact, it’ll allow you to do even more.
What Could You Do With Google Image Search API?
Since all of the features of Google Image Search API are available in the Google Custom Search API, the capabilities of the former are still relevant. One of the major advantages was the capability of finding images at scale based on keywords.
In other words, it worked like regular Google Image Search, but faster and more efficiently. Additionally, downloading all of the found images with corresponding metadata was another major feature.
Image results can also be filtered by size, image type, dimensions, and even usage rights to ensure copyright protection.
Finally, the response format can be optimized as well. Results are always returned in a JSON format. However, the fields and data points can be narrowed down to fit the developer’s exact requirements.
How to Set Up Google Custom Search API as Google Image API
While not as straightforward as previously, setting up Google Custom Search API to replace Google Image API takes just a couple of steps.
First, you’ll need to head over to the Custom Search API page to build a CSE. Scroll down a bit and open a new tab for the Programmable search engine page .
If you don’t have a Google Cloud account, that will also be a great opportunity to create one. Since there’s a free tier for low usage, pick it up for your experiment.
Once you’re in the Programmable search engine page, click on ‘ Add ’ to start creating your custom search engine. In the new screen, select ‘ Search the entire web ’ and toggle ‘ Image search ’ on. Remember to also give your custom search engine a name!
After you’re done, click on ‘ Create ’.
Once the custom search engine has been created, you’ll need an API key. That will be your main way of communication with the Google Image API. You can create one in the Custom Search API page by scrolling down and clicking on ‘ Get a Key ’.
Finally, you’ll also need to enable the Search API in your Google Console . Simply search for Search API, click on ‘ Custom Search API ’, select your project and tap on ‘ Enable ’.
It’s usually wise to restrict API key usage to avoid abuse. However, since this is a tutorial for personal use, that’s not necessary at this point.
Integrating Google Image API into Your Application
The following will depend on your programming language of choice, IDE, and numerous other features. We’ll be showcasing how to retrieve Google image data with Python and JavaScript.
With Python, it’s recommended that you install the requests library for ease of use. To do that, run the following command in your Terminal:
pip install requests
To acquire Google image data, you’ll need to use the following (or a similar) function:
import requests
API_KEY = 'YOUR_API_KEY'
CSE_ID = 'YOUR_CSE_ID'
query = 'sunset beach'
def custom_search(query):
url = 'https://www.googleapis.com/customsearch/v1'
params = {
'q': query,
'cx': CSE_ID,
'key': API_KEY,
'searchType': 'image',
'num': 10
}
headers = {
'User-Agent': 'Mozilla/5.0 (compatible; CustomSearchClient/1.0)'
}
response = requests.get(url, params=params)
if response.status_code == 200:
results = response.json().get('items', [])
return results
else:
print(f"Error: {response.status_code}")
return []
images = custom_search(query)
for image in images:
print(f"Title: {image.get('title')}, Link: {image.get('link')}")
You’ll need to enter two values manually. One of them is the API key for your Image Search API. The other is the custom search engine ID, which can be found in the control panel of the Programmable Search Engine page . You’ll find it by clicking on your CSE.
Note that putting your API keys in plain text in your code is against good cybersecurity practices. We’re only doing that for explanatory purposes.
JavaScript is a bit simpler if you have everything set up. All you’ll need is the CSE ID and API key to use the Image Search API. Simply reuse the code below:
const API_KEY = 'YOUR_API_KEY';
const CSE_ID = 'YOUR_CSE_ID';
const query = 'sunset beach';
async function customSearch(query) {
const url = `https://www.googleapis.com/customsearch/v1?q=${encodeURIComponent(query)}&cx=${CSE_ID}&key=${API_KEY}&searchType=image&num=10`;
try {
const response = await fetch(url);
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
const data = await response.json();
return data.items || [];
} catch (error) {
console.error('Error fetching image results:', error);
return [];
}
}
customSearch(query).then(images => {
images.forEach(image => {
console.log(`Title: ${image.title}, Link: ${image.link}`);
});
});
Advanced Usage and Customization
You’ll likely want more than just a list of titles and links from your Image Search API. There are plenty of areas where you can expand into, ranging from building more complex Image Search APIs to a Google image scraper.
Building a Google image scraper is relatively easy from the previously outlined code. You already get a list of URLs, so, for a Google image scraper, all you need to do is to send a request to the list and retrieve the images.
Alternatively, you can also expand the existing Image Search API to narrow down search results. We’re currently only retrieving up to 10 images, but the number can be adjusted relatively easily (in the URL for JavaScript and in the dictionary for Python).
Take note that the free tier is limited to a small number of requests, and increasing the number of results may quickly increase costs or limit your image search results. To get the most out of the API and perform large-scale image search result retrieval, you’ll need a paid tier.
You can also add various filters to the parameters to change the image search results directly. For example, imgSize, imgColorType, imgType are all common filters you can use to change the retrieval process of your Image Search API.
Note that filters have specific arguments that you need to set for them to work. Image size, for example, accepts icon, small, medium, xlarge, xxlarge, and huge. Be sure to look these up in the documentation to ensure you get the correct image search results.
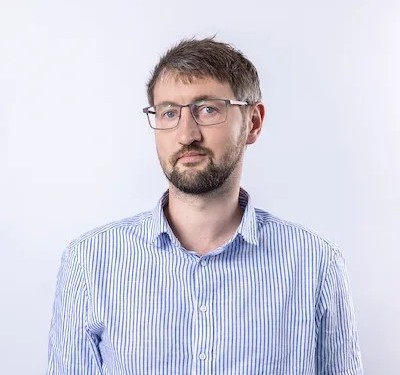
Author
Nerijus Kriaučiūnas
Head of DevOps
With a strong background in system administration, Nerijus has honed his expertise in web hosting and infrastructure management through roles at various companies. As the Head of DevOps at IPRoyal, he oversees product administration while playing a key role in managing residential and ISP proxies. His vast technical expertise ensures streamlined operations across all IPRoyal’s services. When he’s not focused on work, Nerijus enjoys cycling, playing basketball, and hitting the slopes for a ski session.
Learn More About Nerijus Kriaučiūnas