How to Scrape Instagram Data with Python: A Step-by-Step Guide
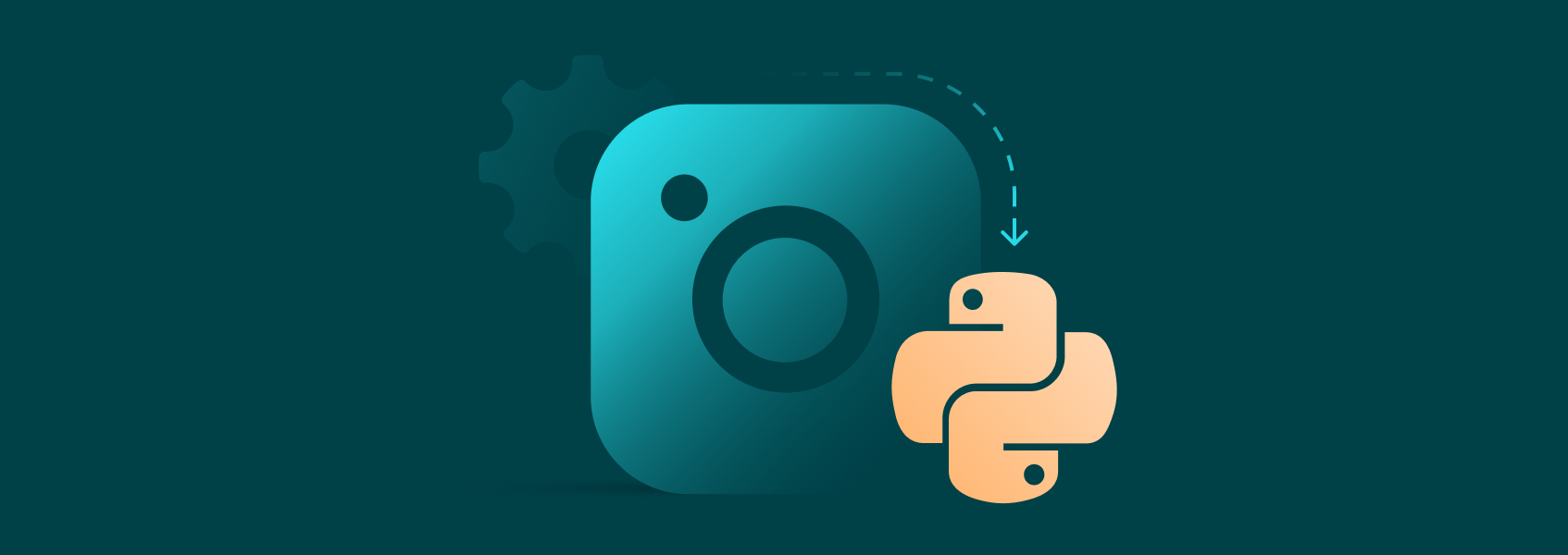
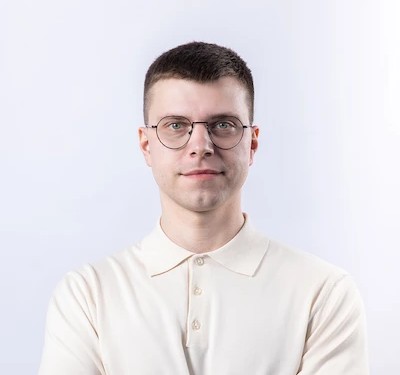
Eugenijus Denisov
In This Article
Key takeaways:
- Instagram scraping works best on public Instagram profiles and must follow the terms of service to avoid legal trouble.
- Tools like Instaloader help you pull Instagram post data, followers, and comments without using the official Instagram API.
- Always store your Instagram data safely and use a trusted Instagram data scraper or Instagram web scraper to avoid being blocked.
If you’re looking to dig into Instagram data without constantly going in circles and wondering about ethical ways to do it, we’ll tell you all about it. We’ll walk you through how to do Instagram scraping using Python.
In this article, you’ll learn how to get data from Instagram profiles, Instagram posts, and even Instagram comments. Here you will find simple solutions whether you’re working on a school project or building an Instagram scraping tool. Let’s get to it.
Is It Legal to Scrape Instagram?
Before jumping into scraping Instagram data, it’s useful to know what’s okay and what’s risky.
Instagram’s Terms of Service say you can’t access the platform in ways that aren’t official and legitimate, like using bots or Instagram scrapers that mimic real users. This makes most types of Instagram scraping against the rules.
That said, there’s a difference between public Instagram data and private Instagram data. Instagram usernames, post counts, follower counts, bios, and Instagram hashtags fall under public data. However, everything else that becomes hidden if someone makes their Instagram profile page private is off-limits.
Scraping public Instagram data is less risky but still not 100% safe. If you make too many requests in a short period, Instagram’s AI might flag your activity. You could get your IP banned or your Instagram account blocked.
If you’re going to scrape Instagram data, make sure to watch out for behaviors like:
- Sending too many requests too fast
- Logging in and out too often
- Trying to scrape Instagram post data in bulk
To lower your chances of getting noticed and blocked even further, you may want to try using an Instagram proxy .
How to Scrape Instagram Profiles, Followers, and Comments
You don’t need to be an expert coder to scrape Instagram profiles and posts, but a little Python know-how will surely help. In this step-by-step section, we will focus on public Instagram profile pages, Instagram posts, and Instagram comments only.
Step 1: Set Up Your Environment
Before you start scraping Instagram data, you need to prepare your workspace. First, install Python 3 on your computer. Then, open an IDE (VS Code or PyCharm works great).
Now, let’s talk about tools. You’ll need:
- Instaloader
A handy Instagram scraper that pulls Instagram data from public profiles.
- Pandas
A library that helps organize and work with Instagram data.
- JSON
A built-in module to save the scraped information in a simple format.
Once you’re ready, open your terminal or command prompt and type this:
pip install instaloader pandas
That’s it. You now have the basic setup to begin Instagram scraping. If you’re curious about more scraping-related insights, check out these helpful web scraping examples that we’ve prepared for you.
Step 2: Scrape Profile Info
Now let’s start with the basics. You’re going to pull public Instagram profile data like the username, bio, number of followers, and post count. This will help you get a quick summary of a user’s Instagram account.
Here’s how it works:
- Load the Instaloader tool
- Choose a public account (let’s say “Natgeo”)
- Ask Instaloader to fetch that user’s profile info
Here’s how the code could look like:
import instaloader
loader = instaloader.Instaloader()
profile = instaloader.Profile.from_username(loader.context, "natgeo")
print("Username:", profile.username)
print("Bio:", profile.biography)
print("Followers:", profile.followers)
print("Posts:", profile.mediacount)
This will print key Instagram data from that profile. It’s a good place to start before moving to more advanced steps.
Step 3: Collect Followers
You can grab the list of followers from any public profile you choose. It shows you all the Instagram usernames that follow the account. However, if the account is private, you’ll only be able to get the number of followers, not a list of them.
Here’s what you can do:
- Use the profile object you already created
- Call a function that gives back all followers
- Loop through them and print their usernames
Here’s the code:
followers = profile.get_followers()
for follower in followers:
print(follower.username)
That gives you a clean list of followers. Be careful not to send too many requests quickly, as Instagram’s systems may flag you for it. It’s best to go slow or use a rotating residential proxy while scraping Instagram data.
Note that you can only extract followers after logging in. Make sure you have the consent or necessary legal support to collect such data.
Step 4: Grab Comments data from Instagram Posts
This step is for you if you want to get Instagram comments from someone’s Instagram posts. Here’s how you can do it:
- Loop through each Instagram post from the profile
- For every post, you collect comments
- Print the comment text data from Instagram and who said it
Here’s the code:
import instaloader
# Initialize Instaloader
L = instaloader.Instaloader()
# Login (optional but required for accessing comments)
USERNAME = 'your_username'
PASSWORD = 'your_password'
L.login(USERNAME, PASSWORD)
# Load profile
profile = instaloader.Profile.from_username(L.context, 'target_username')
# Loop through posts
for post in profile.get_posts():
print("Post:", post.shortcode)
# Fetch comments (requires metadata download)
comments = post.get_comments()
for comment in comments:
print("Comment by", comment.owner.username + ":", comment.text)
That’s how you can pull Instagram post data from comments. Try it out on just a few Instagram posts first so you don’t overload the system.
If you’re looking for more Instagram data or features that could allow you to go deeper with your Instagram scraping efforts, you may want to try the Instagram graph API. It gives you more control, but it’s an official tool and needs tokens.
Instaloader uses the unofficial Instagram API, which is faster to start with and is enough for Instagram scraping beginners.
Step 5: Store the Instagram Data (CSV, JSON, or Database)
You don’t want to just look at your Instagram data in the terminal. You need to save it somewhere safe and easily accessible. There are three main ways to store your Instagram scraping data:
- Save to CSV
You can use Pandas to create a spreadsheet-like file to store your Instagram scraping results.
import pandas as pd
data = {"Username": [], "Bio": [], "Followers": [], "Posts": []}
data["Username"].append(profile.username)
data["Bio"].append(profile.biography)
data["Followers"].append(profile.followers)
data["Posts"].append(profile.mediacount)
df = pd.DataFrame(data)
df.to_csv("profile_data.csv", index=False)
- Save to JSON
This format is great for saving structured Instagram post data in a code-friendly form.
import json
with open("profile_data.json", "w") as f:
json.dump(data, f)
- Save to a database
This is more advanced, but if you’re collecting a lot of Instagram data, a database might be the way to go.
If you’d like to learn more about all things scraping, check out our article on best web scraping practices and get valuable insights for your work.
Conclusion
Now you should know how to set up your tools for Instagram scraping, how to pull data from public Instagram profiles (such as Instagram usernames, followers, and comments), and how to save all that Instagram data for later use.
Just make sure you’re taking Instagram scraping slowly and stay within the ethical boundaries to minimize your chances of getting blocked by Instagram’s systems.
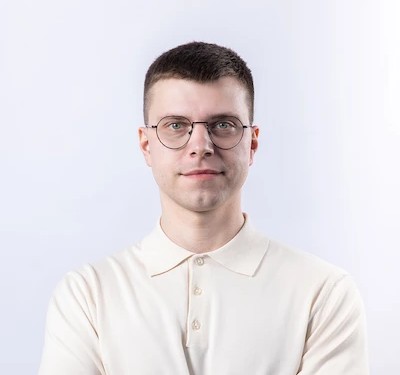
Author
Eugenijus Denisov
Senior Software Engineer
With over a decade of experience under his belt, Eugenijus has worked on a wide range of projects - from LMS (learning management system) to large-scale custom solutions for businesses and the medical sector. Proficient in PHP, Vue.js, Docker, MySQL, and TypeScript, Eugenijus is dedicated to writing high-quality code while fostering a collaborative team environment and optimizing work processes. Outside of work, you’ll find him running marathons and cycling challenging routes to recharge mentally and build self-confidence.
Learn More About Eugenijus Denisov