How to Parse JSON in JavaScript: A Beginner’s Guide
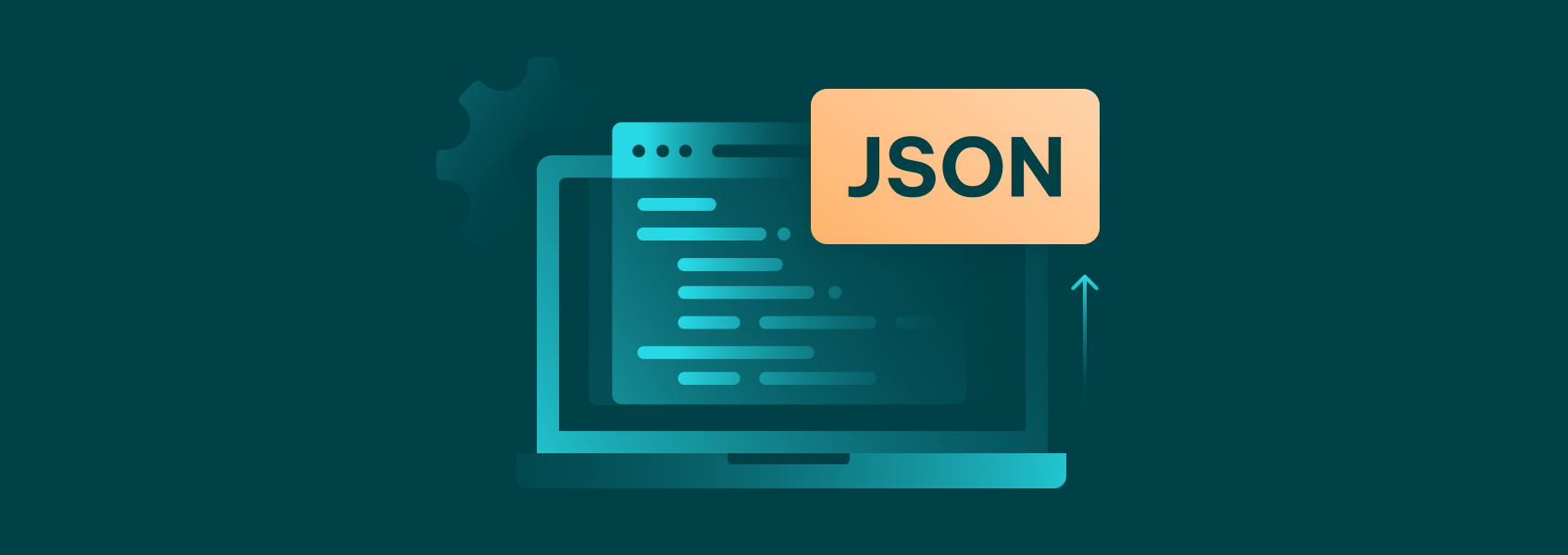
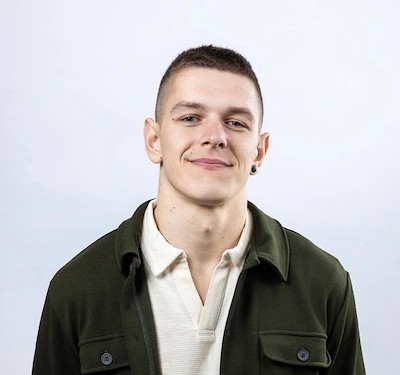
Marijus Narbutas
In This Article
JSON (JavaScript Object Notation) is one of the most popular data storage formats, closely followed by other esteemed structures such as CSV or XML. It’s relatively easy to understand, parse, and work with while also being somewhat lightweight.
It does have its drawbacks, such as lacking data and format verification tools (e.g., schemas). JSON also has no built-in support for comments, so it’s often harder for third-party users to quickly make use of the data structure when compared to some other formats.
What Is JSON?
The JSON format was invented as a way to simplify communication between servers and browsers but it quickly went above and beyond. Many now use it as an alternative to XML, another popular data format that’s widely used for data transfer and communication.
One of the biggest benefits of the JSON format is the combination of human-machine readability. While not as easy to understand as pure text, JSON is still quite easy to read through, and due to its popularity, there’s numerous tools and solutions that can automatically parse the data structure .
There are differences from a JavaScript object, however. Even if they’re named after the same programming language, a JavaScript object is stored within code and represents properties and attributes. A JSON file is simply a data structure that has several requirements to function properly:
- Double quotes around all keys and string values
- Valid data types (string, number, boolean, null, array, object)
- No trailing commas in objects or arrays
- No functions or undefined values
Having the JSON format structured in such a way allows many programming languages to easily parse through the file and extract relevant results. In general, the JSON format is considered language-agnostic, even if it’s named after JavaScript.
Understanding the JavaScript JSON Parse Function
Within JavaScript, JSON parse converts properly formatted JSON files into a JavaScript object, e.g.:
const jsonString = '{"name":"Alice","age":30,"skills":["JavaScript","Python"]}';
const userProfile = JSON.parse(jsonString);
console.log(userProfile.skills[0]);
If you were to run the code, it would output “JavaScript” into the console. While we used a JSON string, you can easily interact with files to convert them to a JSON object.
Note that the JSON.parse command only converts valid JSON, based on the structure. If the file is improperly formatted or structured, you’ll get one of many error messages indicating issues. For example, if you’re missing quotes around properties, you may get the following message:
SyntaxError: Unexpected token a in JSON at position 15
On the other hand, a valid JSON (string or file) will be perfectly converted into associated data types and objects, making the parse function incredibly useful when working with properly formatted JSONs.
How to Parse Local JSON Files in JavaScript
When referring to local files, it’d mean running a server on your machine that has something to be fetched. In such a case, there are at least two options – using JavaScript directly or using Node.js.
With JavaScript, you can use the Fetch API to collect JSON files from the server:
fetch('data.json')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json(); // Parse the JSON from the response
})
.then(data => {
console.log(data); // Use your parsed JSON data here
})
.catch(error => {
console.error('Error fetching or parsing JSON:', error);
});
On the other hand, if you’re using Node.js, you’ll need to use “fs” and the aforementioned JSON.parse to retrieve the file:
const fs = require('fs');
fs.readFile('data.json', 'utf8', (err, jsonString) => {
if (err) {
console.error('Error reading file:', err);
return;
}
try {
const data = JSON.parse(jsonString);
console.log(data);
} catch (err) {
console.error('Error parsing JSON:', err);
}
});
Both options work great for local JSON files and, at times, it’ll be a matter of personal preference or current integration.
JSON.parse vs. JSON.stringify
While they seem closely related and, in fact, use the same API, parse and stringify are used for different purposes. Parse, as we have seen, converts a JSON into a JavaScript object based on the structure, stringify turns a JavaScript object into a JSON string.
In most cases, you’ll want to use stringify for easier data transmission across servers or machines. Turning an object into a JSON string makes it a lot easier to transfer information while the machine on the other end can turn back into an object.
You may consider these two functions as polar opposites. One turns an object into a JSON string, while the other turns the string into a JavaScript object.
Method | Input | Output | Use case |
---|---|---|---|
JSON.stringify() | JavaScript object | JSON string | Data transmission or storage |
JSON.parse() | JSON string | JavaScript object | Data deserialization |
Here’s an example script that makes use of both functions:
const inventory = {
items: [{id: 1, name: "Widget", stock: 45}, {id: 2, name: "Gadget", stock: 12}],
lastUpdated: new Date()
};
const jsonStorage = JSON.stringify(inventory);
console.log('Stored JSON:', jsonStorage);
// {"items":[{"id":1,"name":"Widget","stock":45},{"id":2,"name":"Gadget","stock":12}],"lastUpdated":"2025-02-20T17:33:00.000Z"}
const restoredInventory = JSON.parse(jsonStorage, (key, value) => {
if (key === 'lastUpdated') return new Date(value);
return value;
});
console.log('Restored date type:', typeof restoredInventory.lastUpdated); // object [1][3]
Tips for Working with JSON.parse
While JSON.parse() is relatively self-explanatory, there are several things you can do to increase the likelihood of successful translation:
1. Use a JSON aware editor for manual inspection . Getting color codings and proper visualization of structure will make life a lot easier.
2. Use validators . There’s plenty of tools like JSONLint that can help you catch syntax errors.
3. Employ try-catch blocks . Error containment will make debugging easier, help manage crashes, and improve code robustness.
4. Type handling : using revivers to handle complex data types and convert them to proper objects.
Generally, JSON.parse() is fairly strong at doing its job. Most of your work will be in making sure the JSON you’ve retrieved is properly formatted and implementing some reviver functions to make the most out of it.
Common Problems and How to Fix Them
There are a few problems almost everyone runs into with JSON.parse(): missing quotes, trailing commas, date serialization, circular references, improper decoding.
Missing quotes and trailing commas are two syntax errors that are quite common.
{"name": "Alice", "age": 30,} | A comma after the age integer causes an error. |
{name: "Bob"} | Missing quotes around “name”. |
These two can be fixed through simple edits, which is made even easier using something akin to JSONLint.
Date serialization issues happen when using stringify, as it turns date objects into ISO strings. A reviver function can reverse that.
Encoding issues happen when there are characters that aren’t covered by the decoder (usually, characters that fall outside ‘utf-8’). One of the best ways to ensure these issues don’t happen is to manage encoding before files are written and delivered to ensure that the format remains the same.
You can also enforce encoding in the retrieval function (not in JSON.parse() as it’s not supported).
Finally, circular references happen when objects refer to themselves. Deletion or manual modification is your best bet.
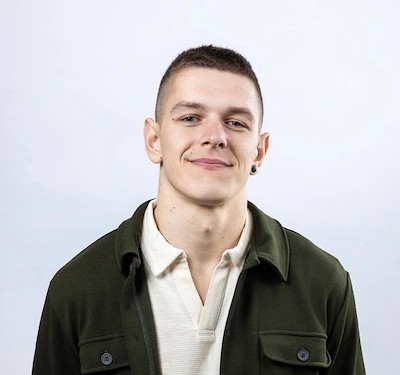
Author
Marijus Narbutas
Senior Software Engineer
With more than seven years of experience, Marijus has contributed to developing systems in various industries, including healthcare, finance, and logistics. As a backend programmer who specializes in PHP and MySQL, Marijus develops and maintains server-side applications and databases, ensuring our website works smoothly and securely, providing a seamless experience for our clients. In his free time, he enjoys gaming on his PS5 and stays active with sports like tricking, running, and weight lifting.
Learn More About Marijus Narbutas