What Is ParseError? Python Edition
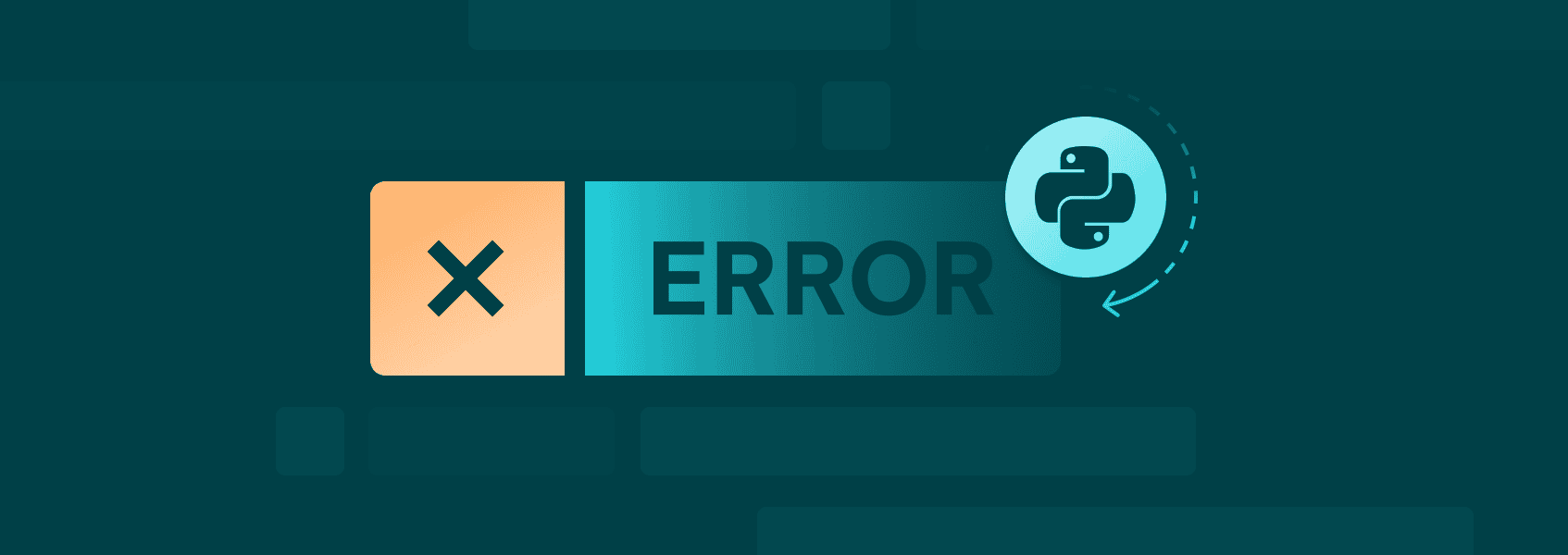
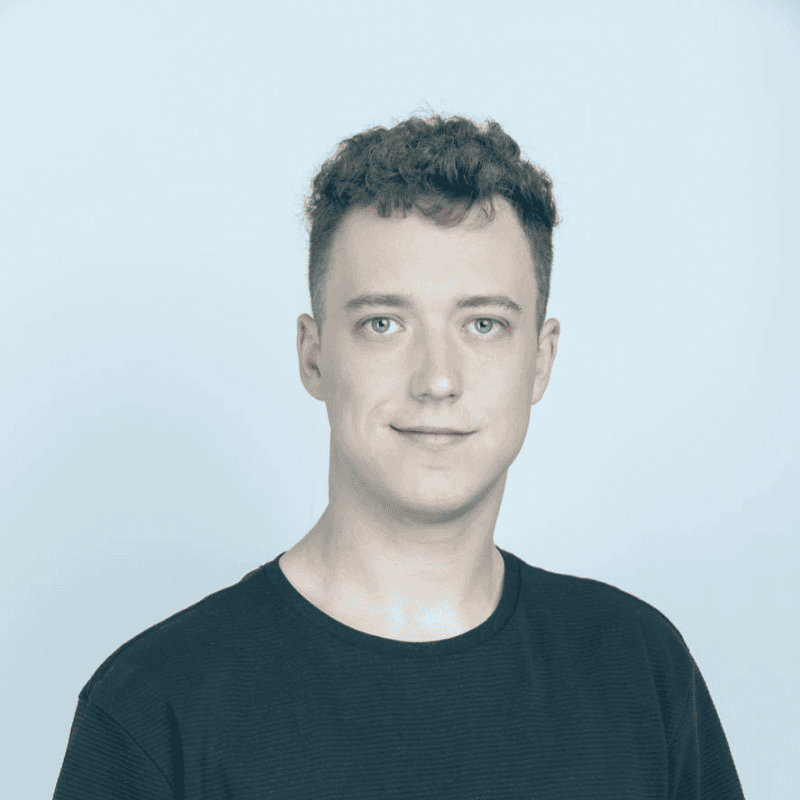
Vilius Dumcius
Last updated -
In This Article
Every programming or scripting language has a predefined way code should be written. If a piece of code does not match those requirements, the programming or scripting language’s interpreter will throw a parse error.
In Python, technically, there isn’t an inbuilt parse error. Instead, most of what other languages would consider a parse error, Python considers them to be a syntax error. As such, if there’s a parse error, Python will throw out something like this:
SyntaxError: invalid syntax
What Are Parsing Errors?
Parsing is the process by which a computer program analyzes code to ensure that it conforms to set programming language rules. The task is usually performed by an interpreter or compiler.
An interpreter breaks down all written code into smaller chunks (tokens) and then validates them against the predefined rules. There are several layers of validation:
- Lexical analysis
Breaks code down into the smallest possible meaningful chunks (tokens). They may be keywords, operators, identifiers, and literals, among many other tokens.
- Syntax analysis (parsing)
Each token sequence is checked against the grammar rules of the programming or scripting language. Parsing errors occur at this stage.
- Semantic analysis
Checks various additional features of the code, such as whether types are correctly assigned and if there are no unusual sequence breaks.
- Execution
Runs the parsed code.
Most programming languages, Python included, go through an identical or extremely similar process. Python only calls the error message “syntax error” instead of “parsing error”.
As such, the definition of a parsing or syntax error is an error that occurs when the code written by the programmer does not conform to the rules or grammar of the programming language.
Note that parsing in programming languages is not the same as data parsing . The latter is mostly working with various data types to structure and convert them into something more easily understandable.
Common Python Syntax Errors
While there are uncountably many possible parse errors, there are at least a few that are most common. Most of these are simple mistakes like typos.
Misusing the Assignment Operator
The assignment operator (“equals to” sign) has two applications that are commonly misused in Python:
- A single assignment operator (e.g., “x = 5”)
Using the assignment operator once attaches a specific value to an object or variable. In the example outlined above, x is assigned the integer value of 5.
- Double assignment operator (e.g., “x == 5”)
Compares the values of objects and determines if the values of two objects are equal. For example: “a = 5”; “b = 5”; “a==b” would be true as both objects have the same value.
It’s extremely easy to make a simple mistake when using the double assignment operator. If only a single one is typed in, the object will be assigned a new value, which can cause parse errors, cause the code to behave unpredictably, or even cause Python exceptions.
Sample code that causes the Python interpreter to throw a syntax error:
my_func = lambda x == 10: x + 1
Using Parentheses, Brackets, and Quotes Improperly
Misusing parentheses, brackets, and quotes is so common that most modern IDEs nowadays automatically include both sides of the expression (e.g., []) once you type in the left-hand side sign.
Failing to properly close parentheses, brackets, or quotes will almost always cause parse errors as the Python interpreter will consider all of the following code as included in the parentheses. Alternatively, if there’s no closing sign in the remainder of the code, that will also always cause parse errors.
Sample:
print("Hello, world!"
Missing Comma
A missing comma is also one of the most common Python syntax errors. While the Python interpreter doesn’t require you to finish every line of code with a semicolon or comma, there are cases where the latter is necessary.
These are usually lists, tuples, dictionaries, some ways of importing libraries, some string formatting options, etc.
Sample:
my_list = [1 2 3 4]
Misspelling Keywords
Python, like all programming languages, has a specific set of predefined keywords. Some of the most well-known ones are “if”, “then”, “else”, “for”, “while”, and several others. Each of these has to be typed in exactly the same way every time. A typo inevitably leads to a syntax error message.
Sample:
iff True:
print("This will cause a syntax error.")
Handling Python Exceptions
Resolving syntax errors in Python is relatively simple if you use any modern IDE. Most of them have advanced syntax validation systems, which will inform you about any potential errors.
For example, the IDE might mark any areas in red where it predicts you made a syntax error. If that doesn’t work, executing the code will provide you with an error message. Usually, it’ll even state the line and point to the exact location where the syntax error occurred.
One of the best practices for errors is usually handling Python exceptions. Syntax errors can also be handled, but many of them will occur at the development stage instead of the execution stage.
Handling syntax errors is usually used in two cases. First, whenever users have input. Handling syntax errors in that case can provide valuable information to the user. Another option is to handle syntax errors when executing dynamic code (such as with “exec” or “eval” functions).
Here’s how you could handle syntax errors with dynamic code:
user_code = "print('Hello World!')\nprint(2 + )"
try:
exec(user_code)
except SyntaxError as e:
print(f"Syntax error in user code: {e}")
Note that executing the code will print the error message instead of using the default one.
Generally, you’ll be using the “try” and “except” functions to handle exceptions. Syntax errors are no different in that regard.
Conclusion
Solving parsing errors in Python is mostly an effort of looking over your code to find missing commas, parentheses, or misspelled words. In more advanced solutions, such as where users have a lot of input or code is dynamically executed, you may want to use “try” and “except” to handle syntax errors.
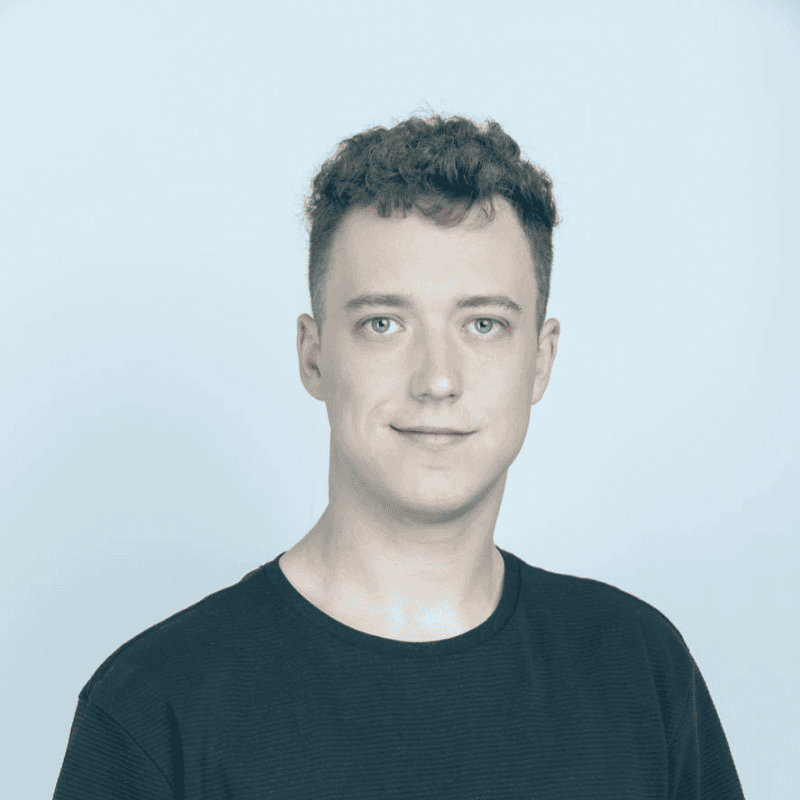
Author
Vilius Dumcius
Product Owner
With six years of programming experience, Vilius specializes in full-stack web development with PHP (Laravel), MySQL, Docker, Vue.js, and Typescript. Managing a skilled team at IPRoyal for years, he excels in overseeing diverse web projects and custom solutions. Vilius plays a critical role in managing proxy-related tasks for the company, serving as the lead programmer involved in every aspect of the business. Outside of his professional duties, Vilius channels his passion for personal and professional growth, balancing his tech expertise with a commitment to continuous improvement.
Learn More About Vilius Dumcius