Python Cache: Understanding Caching Basics
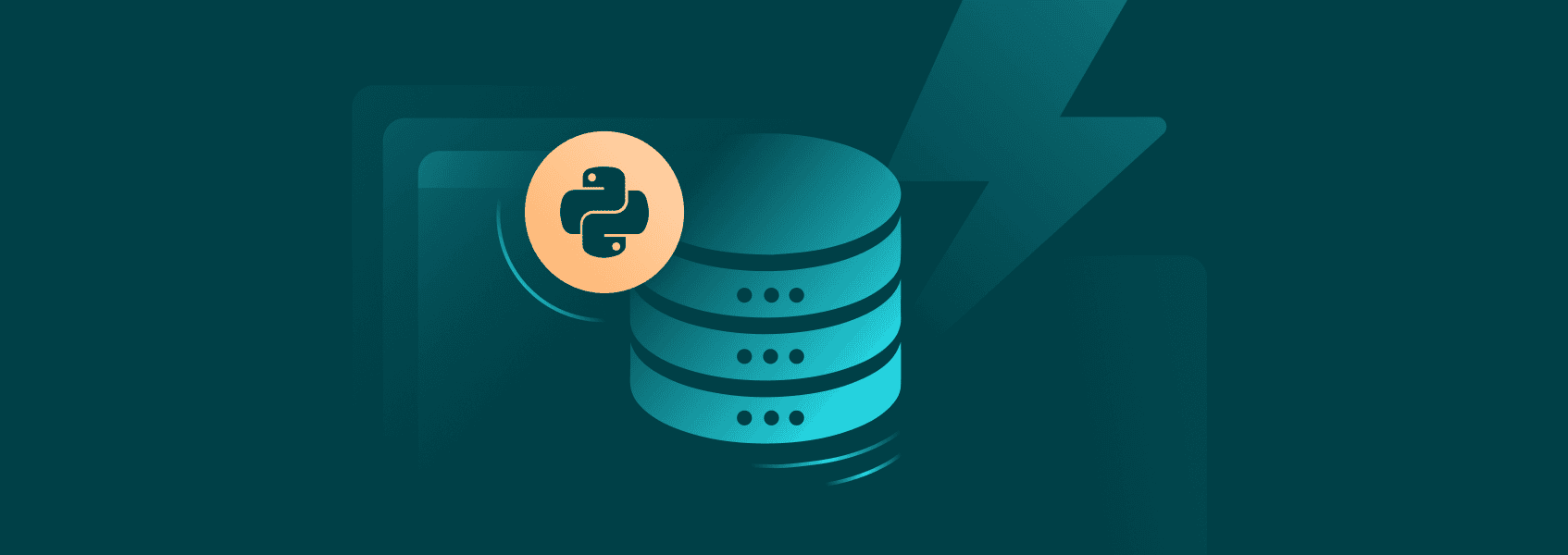
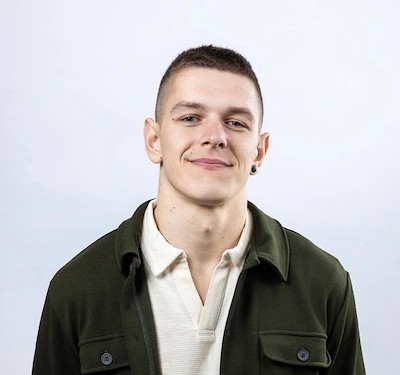
Marijus Narbutas
In This Article
A cache is a previously stored snapshot of data, often used to improve performance and resource efficiency. For example, if you visit the same website twice in short succession, it’ll store the data of the first visit and may serve the information it stored on the second visit.
Using cache in such a way avoids having to redownload all the files and improves loading speed. In software development, various caching strategies are used to ensure that as much data and performance is saved while the usability and experience of the user doesn’t suffer.
Caching strategies are widely used in Python for the same purposes. Almost every advanced application will have some form of cache to improve performance, so including one is a modern necessity.
What Is Caching in Python?
Caching is a storage and performance strategy that allows developers to avoid unnecessary computation, API calls, or other processes that may slow down the application’s intended purpose. Instead of executing code or externally retrieving data, an application with a cache can assess the previous results and reuse them for its next step.
Using caching in Python can bring several benefits, such as quicker execution times and reduced computational load. Overall, a properly implemented caching strategy will greatly improve performance.
A simple example of a cache is if a value needs to be calculated once and isn’t likely to change frequently. Instead of calling the calculations each time, the cached value can be stored and reused for further actions.
Many developers use caching in Python almost unintentionally. Storing values in dictionaries, for example, can be considered caching if the data takes some time to calculate. Basic caching strategies are simply good programming practices, especially if recursive functions are used.
There are plenty of different caching strategies that can be implemented intentionally, however.
Ways to Cache in Python
Manual Caching in Python
It’s likely that every developer has used manual caching at least once, even if unintentionally. Manual caching is the process of storing values in objects like dictionaries and reusing them later.
A simple example of such Python caching:
# Manual caching example
cache = {}
def expensive_function(x):
if x in cache:
return cache[x]
else:
result = x * x # Hypothetical expensive calculation
cache[x] = result
return result
# Usage
print(expensive_function(10)) # Caches result of 10*10
print(expensive_function(10)) # Retrieves from cache
Manually building a caching mechanism could be beneficial for small amounts of data to store and retrieve.
Such a caching method is overly simplistic, however, and there are many more efficient methods available, mostly through various libraries such as lru_cache available through the functools module.
Using lru_cache() for Caching
Python’s functools module offers a decorator, lru_cache(), that implements a specific strategy called “Least Recently Used” (LRU). As maximum cache size is reached, LRU removes the least recently used data structures from the cache.
One of the major benefits to using LRU caching strategy is that it greatly simplifies code while also being quick and efficient. You don’t need to set up some cache dictionary object yourself – lru_cache() does everything on its own.
from functools import lru_cache
@lru_cache(maxsize=128)
def expensive_function(x):
return x * x
# Usage
print(expensive_function(10)) # Caches result
print(expensive_function(10)) # Retrieves from cache
Note that you can set your own maximum size with the cache decorator (@lru_cache), however, making it needlessly large does have some drawbacks. Namely, memory usage increases with cache size, which can cause paging (data being moved to hard storage), dramatically slowing down performance.
Third-Party Libraries for Caching
Python caching doesn’t have to rely on the *functools *module exclusively. There are plenty of third-party libraries that will do the job just fine or maybe even better than the inbuilt Python caching methods.
Two good examples are cachetools and requests-cache . The former is a great all-round alternative to the regular lru_cache method while the latter is better for HTTP requests and can complement a Python client library greatly.
There are plenty more of them out there, so you can always find something that will fit your needs best.
When to Use Caching in Python
There’s often little reason to avoid caching unless you’re writing a small project or it’s a one-and-done task. Any larger application, website, API, or any other project can benefit from caching.
Primarily, caching will be heavily used in three areas:
- Web (API) development
Repeated queries are common, so caching database data will significantly improve overall performance.
- Data science
Caching some data that’s used for calculations can greatly reduce computation times.
- Memory-intensive functions
Any application or function that uses a lot of memory can benefit from caching, so that will include most advanced software development.
Caching, in general, is a great tool that can rarely lead you astray unless enormous sizes are dedicated to it. As long as you don’t run into paging issues or cache management problems, it’s a wise idea to use at least some of that.
Caching Challenges in Python
While caching is truly beneficial in many scenarios, there are a few circumstances that make such applications difficult. One of the greatest challenges is cache invalidation wherein the stored data no longer matches the real world.
Avoiding cache invalidation is often considered one of the biggest programming challenges and, at some level, it’s almost bound to happen. So, the updates and recalculations have to be carefully considered to avoid invalidation issues.
Additionally, there are various cache management challenges such as increased memory load and undue size. Both of these challenges lead to performance issues down the road, making caching significantly less valuable.
Managing caches and avoiding the challenges is an extremely complex topic that cannot be fully covered in a mere chapter of a blog post. It’s recommended that you always keep learning about new methods and ways to manage caching.
How Long Does Python Cache Last?
All caching methods have a set duration, depending on the method used. Manual caches, for example, are fully managed by the developer , so their duration can be modified as seen fit.
LRU caches, on the other hand, last until the maximum size limit is reached, then the last recently used data is removed to make space for new information. As such, the duration is tied to frequency of storage.
Finally, there are duration-based caches that last until a set amount of time elapses. They are then wiped clean, and new data is stored after a request or calculation.
As such, there’s no single answer to how long a cache can last as there are multiple methods to manage, store, and clean them.
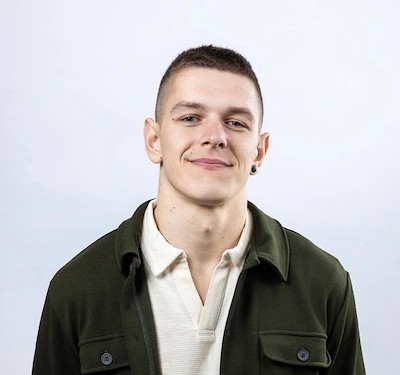
Author
Marijus Narbutas
Senior Software Engineer
With more than seven years of experience, Marijus has contributed to developing systems in various industries, including healthcare, finance, and logistics. As a backend programmer who specializes in PHP and MySQL, Marijus develops and maintains server-side applications and databases, ensuring our website works smoothly and securely, providing a seamless experience for our clients. In his free time, he enjoys gaming on his PS5 and stays active with sports like tricking, running, and weight lifting.
Learn More About Marijus Narbutas