Mastering the Python GitHub API: A Practical Guide for Developers
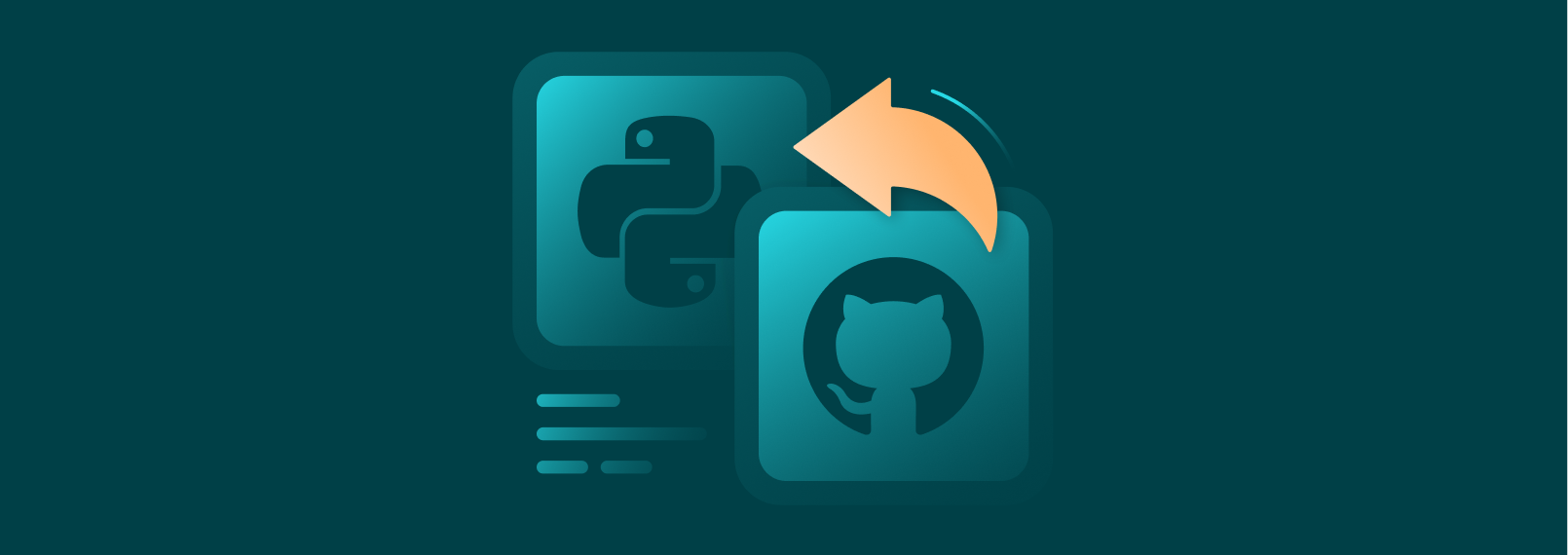
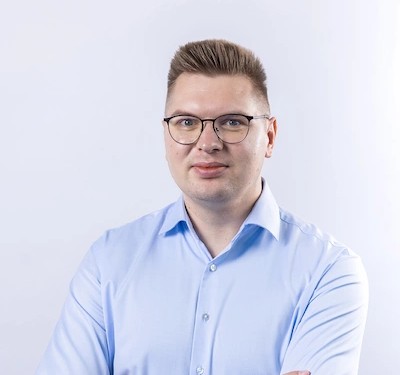
Justas Vitaitis
In This Article
Key takeaways:
- Automate tasks like repository management and issue handling with the REST API.
- Use personal access tokens securely stored in environment variables.
- Automate repository creation, collaborator management, and issue tracking with Python.
- Implement error handling and use caching/pagination for efficient API use.
- Use HTTPS, avoid hardcoding tokens, and monitor API rate limits.
GitHub has become an essential tool for developers. It offers a platform to manage code, collaborate with teams, and track project progress. The GitHub API allows developers to interact programmatically with GitHub , automating tasks like repository management, issue tracking, and user data retrieval.
This way, developers can streamline workflows and enhance productivity.
Python, with its simplicity and powerful libraries, is an excellent choice for interacting with the GitHub API. Its ease of use makes it ideal for beginners, while its flexibility allows for complex automation and integration.
In this guide, we’ll walk you through the essential steps to get started with the GitHub API using Python.
What Is the GitHub API?
The GitHub API is a set of web services that allow developers to interact programmatically with GitHub. It provides endpoints for interacting with data on GitHub, such as repositories, users, issues, pull requests, and much more.
The GitHub API enables you to automate tasks, integrate GitHub functionality into your applications, or extend GitHub’s features in various ways.
There are two main versions of the GitHub API:
REST API
The REST API accomplishes CRUD operations through resource-based URLs that support Create, Read, Update, and Delete functions. The API functions through standard JSON requests, which implement GET, POST, PUT, and DELETE HTTP methods. It provides data output in JSON structure.
GraphQL API
GraphQL API provides users with a flexible solution because it enables them to request particular fields within each request. The API structure reduces over-fetching, requiring users to know query structures thoroughly.
Differences Between REST and GraphQL GitHub API
- REST API is best suited for simple operations and is more intuitive for beginners.
- GraphQL API is ideal for advanced scenarios requiring performance optimization by reducing multiple API requests.
For this guide, we’ll focus on the REST API due to its ease of use and accessibility for beginners.
Setting Up Python and GitHub API Authentication
To interact with the GitHub API using Python, you’ll need to install the required libraries:
pip install requests PyGithub
requests: Allows sending HTTP requests to APIs. PyGithub: Provides an object-oriented wrapper for the GitHub API, simplifying interactions.
Generating a GitHub Personal Access Token for Authentication
Authentication requires a Personal Access Token (PAT) to access the GitHub API securely.
- Go to GitHub, click your profile photo, and select ‘ Settings’.
- Navigate to ‘ Developer Settings’ > ‘ Personal Access Tokens’.
- Choose ‘Generate new token’, assign it a name, set its expiration, and select the necessary scopes.
- Copy and securely store the token, as it cannot be retrieved later.
Users can decide between full repository permission or individual selection under ‘Repository access’ on the GitHub platform. The best approach for security reasons is to control token access through specific selected GitHub repositories.
Choose ‘Generate token’ from the screen to finalize your token configuration. The new token appears right away on GitHub, so take a cautionary note to save it for future use because retrieval later is impossible.
All operations related to personal access tokens must be performed while ensuring security. You should avoid storing tokens directly within your scripts or placing them in GitHub repositories even when they are private because token exposure is possible.
All tokens need proper storage in environment variables, or GitHub Actions should use encrypted secrets in the repository configuration.
Periodically inspect and manage your active tokens to deactivate every unused and endangered access token you possess. When implementing these best practices, your secure and efficient GitHub API connection becomes possible, creating a responsible management approach to your GitHub environment.
Making API Requests with Python
GitHub API enables developers to execute different actions through Python through HTTP requests and the PyGithub library. The three principal API operations include fetching user details and listing GitHub repositories while creating repositories, which forms the third core operation.
Fetch User Details
Any GitHub user can be searched using a GET request directed at the /users/{username} endpoint. The desired GitHub username must replace {username} to retrieve information about the user, including a bio and their public repositories and profile details.
The following query demonstrates how to retrieve user information:
python library
import requests
# Define the target GitHub username
username = "your_github_username" # Replace with the desired GitHub username
# Define the API endpoint URL
url = f"https://api.github.com/users/{username}"
try:
# Send a GET request to fetch user data
response = requests.get(url)
response.raise_for_status() # Raise an exception for HTTP errors
user_data = response.json()
# Print user details
print(f"Username: {user_data['login']}")
print(f"Bio: {user_data['bio']}")
print(f"Public Repositories: {user_data['public_repos']}")
except requests.exceptions.RequestException as e:
print(f"Error retrieving user data: {e}")
List Repositories
To list all public repositories of a user, you can send a GET request to the /users/{username}/repos endpoint. This returns a JSON array containing all repositories with relevant metadata.
Here’s an example of listing repositories:
import requests
# Define the target GitHub username
username = "your_github_username" # Replace with the desired GitHub username
# Define the API endpoint URL for repositories
url = f"https://api.github.com/users/{username}/repos"
try:
# Send a GET request to fetch repositories
response = requests.get(url)
response.raise_for_status()
repos_data = response.json()
print(f"Public repositories for {username}:")
for repo in repos_data:
repo_name = repo['name']
repo_desc = repo.get('description', 'No description provided')
print(f"- Name: {repo_name}")
print(f" Description: {repo_desc}")
except requests.exceptions.RequestException as e:
print(f"Error retrieving repository data: {e}")
Create a Repository
To create a new repository programmatically, you can use the PyGithub library, which provides an object-oriented interface to interact with GitHub resources. The create_repo() method allows users to create a repository and configure its description and visibility.
Here’s an example of creating a new repository using PyGithub:
from github import Github
# Replace "YOUR_ACCESS_TOKEN" with your actual personal access token
g = Github("YOUR_ACCESS_TOKEN")
# Get the authenticated user
user = g.get_user()
# Define repository details
repo_name = "your-new-repository-name" # Replace with your desired repository name
repo_description = "A description for your new repository" # Optional description
# Create the repository
try:
repo = user.create_repo(repo_name, description=repo_description)
print(f"Repository '{repo.name}' created successfully! URL: {repo.html_url}")
except Exception as e:
print(f"Error creating repository: {e}")
Automating Tasks with GitHub API
Automating repetitive tasks using the GitHub API enhances workflow efficiency by simplifying repository management, issue creation, and pull request handling. With Python and the PyGithub library, developers can perform tasks such as creating repositories, adding collaborators, automating issue creation, and assigning issues dynamically.
Repository Management with PyGithub
Creating a new repository usually requires making POST requests to the /user/repos endpoint for authentication purposes while supplying details such as repository name, repo description, and privacy settings.
You can establish a new repository while offering an optional name repo description. Using PyGithub, you can programmatically create, modify, and delete repositories with minimal effort. Below are examples of key repository management tasks:
1. Creating a Repository
To create a repository, use the create_repo() method to set a repository name, description, and visibility.
from github import Github
# Replace "YOUR_ACCESS_TOKEN" with your actual personal access token
g = Github("YOUR_ACCESS_TOKEN")
# Get the authenticated user
user = g.get_user()
# Define repository details
repo_name = "your-new-repository-name" # Replace with your desired repository name
repo_description = "A description for your new repository" # Optional description
# Create the repository
try:
repo = user.create_repo(repo_name, description=repo_description)
print(f"Repository '{repo.name}' created successfully! URL: {repo.html_url}")
except Exception as e:
print(f"Error creating repository: {e}")
2. Adding Collaborators to a Repository
You can automate adding collaborators to your repositories using the add_to_collaborators() method. This method grants access to specific users, helping to streamline team collaboration.
from github import Github
# Replace "YOUR_ACCESS_TOKEN" with your actual personal access token
g = Github("YOUR_ACCESS_TOKEN")
# Get the repository object
repo = g.get_repo("your_username/your_repository") # Replace with your repository details
# Define the collaborator's GitHub username
collaborator_username = "collaborator_username" # Replace with the collaborator's username
# Add the user as a collaborator
try:
user_to_add = g.get_user(collaborator_username)
repo.add_to_collaborators(user_to_add)
print(f"Collaborator '{user_to_add.login}' added to the repository.")
except Exception as e:
print(f"Error adding collaborator: {e}")
#### 3. Deleting a Repository
Deleting repositories using PyGithub is irreversible, making it essential to proceed cautiously.
```python
from github import Github
# Replace "YOUR_ACCESS_TOKEN" with your actual personal access token
g = Github("YOUR_ACCESS_TOKEN")
# Get the repository object
repo = g.get_repo("your_username/your_repository") # Replace with the repository to delete
# Delete the repository
try:
repo.delete()
print(f"Repository '{repo.name}' deleted successfully.")
except Exception as e:
print(f"Error deleting repository: {e}")
Automating Issue Management
GitHub API allows developers to automate the creation of issues and manage them dynamically. Automating issue creation can be useful when external systems detect errors or when certain events trigger new tasks.
1. Creating Issues Automatically
You can automate issue creation using the create_issue() method to streamline task reporting and bug tracking.
from github import Github
# Replace with your personal access token and repository details
g = Github("YOUR_ACCESS_TOKEN")
repo = g.get_repo("your_username/your_repository")
# Define issue details
issue_title = "New bug reported from external system"
issue_body = "Details of the bug from the external system go here..."
# Create the issue
try:
issue = repo.create_issue(title=issue_title, body=issue_body)
print(f"Issue '{issue.title}' created successfully.")
except Exception as e:
print(f"Error creating issue: {e}")
2. Assigning Issues Based on Conditions
You can dynamically assign issues to specific team members based on certain criteria using the edit() method. This ensures that issues are directed to the appropriate person automatically.
from github import Github
# Replace with your personal access token and repository details
g = Github("YOUR_ACCESS_TOKEN")
repo = g.get_repo("your_username/your_repository")
# Define the issue number and the assignee's username
issue_number = 123 # Replace with the issue number
assignee_username = "developer_username" # Replace with the username to assign
# Assign the issue to a user
try:
issue = repo.get_issue(number=issue_number)
assignee = g.get_user(assignee_username)
issue.edit(assignees=[assignee])
print(f"Issue #{issue.number} assigned to '{assignee.login}'.")
except Exception as e:
print(f"Error assigning issue: {e}")
Security Considerations
Your personal access tokens need absolute protection whenever you access the GitHub API. Do not place your personal access tokens inside version control systems, especially in private repos, and never write them directly into scripts.
The secure storage of tokens must be done through environment variables and specialized secret-management tools. You should only authorize permissions relevant to daily operations and withdraw unused or compromised tokens through regular reviews.
Protecting Your GitHub Account and Communications
You must always use HTTPS ( https://api.github.com/ ) to connect with the GitHub API because it enables secure encryption of sensitive data during transmission.
Protecting your overall account requires complex passwords with a unique combination that you store in a reputable password manager combined with 2FA implementation, regular reviews of unused third-party application access control, and constant phishing threat awareness.
Integrating GitHub API into Development Workflows
Using GitHub API automation improves teamwork productivity by making project issue management faster while maintaining consistent practices across all projects. Through the API, developers can easily connect GitHub to their development tools, including Jira or Trello integration and event notifications through Slack or Discord, along with CI/CD pipeline triggering and valuable project analytic data sharing.
Developers must thoroughly examine GitHub REST API and PyGithub library documentation to implement all possible capabilities. Alerting developers to new updates, best practices, and advanced features occurs through following the GitHub Developer Blog to achieve maximum potential in development environments.
Common GitHub API Errors and How to Fix Them
When interacting with the GitHub API, developers may encounter errors that can disrupt their workflow. Understanding these errors and applying best practices can ensure smooth and secure API interactions.
Authentication Errors (401 Unauthorized)
Authentication errors occur when an invalid or expired Personal Access Token (PAT) is used or if the token lacks the required permissions. Double-check that your token is active, has the correct scopes, and is included correctly in the request headers. For PyGithub, use:
g = Github("YOUR_ACCESS_TOKEN")
Ensure the token is stored securely in environment variables or secret managers to prevent exposure.
Rate Limit Errors (403 Forbidden)
GitHub enforces rate limits to prevent excessive API usage. Unauthenticated requests have lower limits, while authenticated requests offer higher limits. To monitor your usage and remaining limit, query the /rate_limit endpoint:
import requests
response = requests.get('https://api.github.com/rate_limit', headers={'Authorization': 'token YOUR_ACCESS_TOKEN'})
print(response.json())
If you approach the limit, implement caching strategies or introduce delays between requests to prevent exceeding the limit.
Resource Not Found Errors (404 Not Found)
A 404 error suggests that the requested user, repository, or endpoint is invalid or does not exist. Verify the URL, check for typos in usernames or repository names, and ensure that private repositories or resources require proper authentication.
Best Practices for Efficient GitHub API Usage
To optimize API performance and maintain security, follow these practices:
- Authenticate API requests: Use a personal access token with fine-grained permissions.
- Paginate large responses: Break down responses into manageable pages to handle large datasets efficiently.
- Avoid hardcoding tokens: Store tokens in environment variables and never expose them in source code.
- Monitor API usage: Regularly check rate limits and optimize API calls to prevent interruptions.
- Use HTTPS for secure communication: Always use https://api.github.com/ to protect sensitive data.
By adhering to these best practices and handling errors effectively, developers can ensure secure, optimized, and efficient interaction with the GitHub API.
Error Handling and Optimization
It is necessary to build solid error management structures within GitHub API interactions to handle network failures, authentication errors, resource-not-found errors , and rate limit errors. Python try-except blocks successfully manage exceptions that occur in code operations.
Response.raise_for_status() from the requests library detects HTTP errors, including 401 Unauthorized and 404 Not Found cases. Users of PyGithub can handle errors specifically through RateLimitExceededException and BadCredentialsException exceptions.
The implementation of optimization methods, which include caching along with pagination, maintains equal importance. The requests-cache library helps reduce API calls, enhancing system speed while upholding rate limit protocols from the requests library.
Processing data page-by-page through the Link headers enables efficient handling of paginated responses, stopping your resources from overloading.
Through its APIs, GitHub guards resources by implementing rate limits that provide higher limits for authenticated users. Monitoring your rate limit status through GitHub's /rate_limit endpoint alongside authentication caching and pagination strategies ensures reliable and smooth API interactions.
Conclusion and Next Steps
So here, you have mastered the essential skills of using Python to interact with the GitHub API. Through your learning, you became proficient in Personal Access Token (PAT) authentication and API data retrieval and automated the creation of repositories with manager functions for collaborators and issue resolution. Now you know about error resolution techniques, secure API utilization methods, and effective best practices.
The latest updates alongside best practices and new features become available through regular blog visits at GitHub Developer Blog and by consulting official GitHub API documentation.
The mastery of GitHub API provides developers with capabilities to enhance project development by implementing smooth integration between development workflows and improving repository management, consequently enhancing efficiency and collaboration.
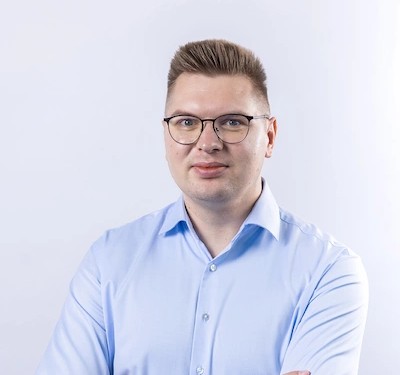
Author
Justas Vitaitis
Senior Software Engineer
Justas is a Senior Software Engineer with over a decade of proven expertise. He currently holds a crucial role in IPRoyal’s development team, regularly demonstrating his profound expertise in the Go programming language, contributing significantly to the company’s technological evolution. Justas is pivotal in maintaining our proxy network, serving as the authority on all aspects of proxies. Beyond coding, Justas is a passionate travel enthusiast and automotive aficionado, seamlessly blending his tech finesse with a passion for exploration.
Learn More About Justas Vitaitis