Python Syntax Errors: What They Are and How to Fix Them
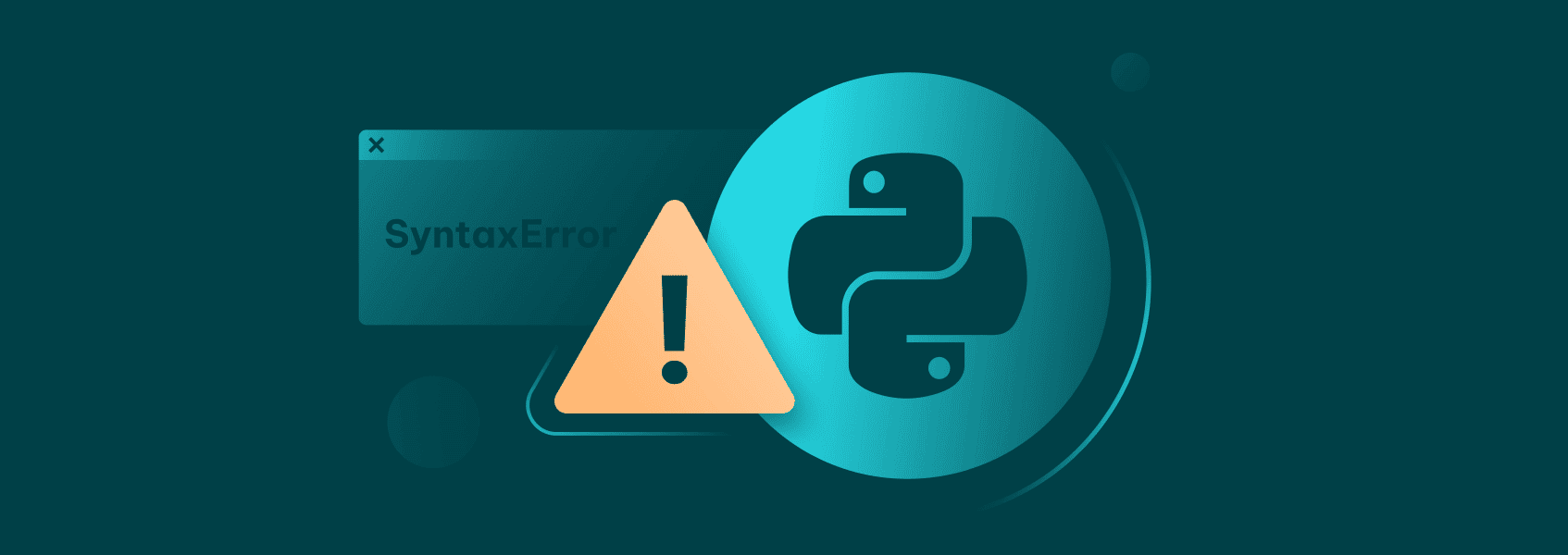
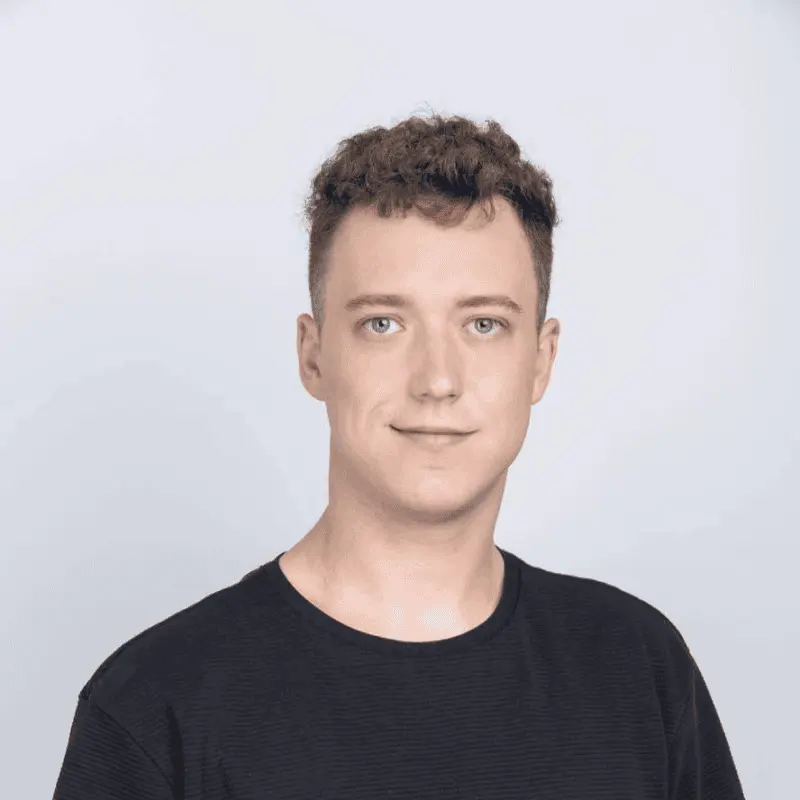
Vilius Dumcius
In This Article
While syntax plays an important role in natural language, it takes the crown in programming. Every programming language has its own unique syntax, and it has to be followed to a T, as the interpreter may be incapable of running any program that has an invalid syntax.
The syntax is close to grammar in natural languages as it defines the rules and structure code must follow. Some programming languages may be a little more lenient in enforcing these rules and attempt to resolve syntax errors.
The Python interpreter is one of the strict ones. Python syntax errors will raise an exception and fail to run any code. So, you’ll need to correct any syntax errors if you want to run your program.
What Are Syntax Errors in Python?
Syntax errors are any mismatch between the way code is written and the way it should be as defined by the Python interpreter. Attempting to run code that has Python syntax errors will raise an exception.
In Python, syntax is of utmost importance as it’s a programming language that has no leniency for it. However, Python is also considered a relatively easy-to-learn language with quite a simple syntax, so fixing most errors is often easy.
Additionally, syntax errors in Python raise an exception that outputs a specific error type. In the output, you’ll find the offending line and the interpreter’s expectations. As such, there’s instantly some guidance into what syntax rules were not upheld and how to fix them.
Finally, it’s important to separate syntax from good programming practices such as Zen of Python . Best practices in programming make use of various techniques such as line breaks, variable naming strategies, and many other methods to make code easier to understand and read for humans.
Even if you break every good programming practice but don’t run into any syntax errors, your code will run flawlessly. It’ll likely be extremely difficult for a human to read and understand but the interpreter will not be faced with any issues.
What Is an Example of a Syntax Error in Python?
Let’s take some common Python syntax errors to showcase. Common mistakes include missing parentheses, colons, and a single quotation mark (instead of two). Here’s an example:
if True
print("Python is cool")
“True” is a Python keyword which represents a boolean value. If paired with a conditional (if), it will always proceed to the “then” part of the statement. So, on the face of it, Python should infinitely print “Python is cool”.
Yet, if you were to run the code, Python would raise a syntax error. There will be a small arrow (caret points) that showcases the particular spot of the code snippet. In this case, it’s “if True” as there’s a missing colon.
Take note, however, that the location indicated is where the interpreter first noticed the issue. There may be cases where the error occurred in a different spot.
Missing colons are among the most common mistakes. Another common mistake that’s sometimes a bit harder to grasp and fix is the assignment operator misuse.
x = 5
if x = 5
print("Python is cool")
At first glimpse, there shouldn’t be any invalid syntax errors. Unfortunately, there’s a great degree of difference between a single “equals to” sign (“=”) and a double “equals to” sign (“==”).
A single “equals to” sign is an assignment operator. It connects the left and right sides of the equation and assigns the stated values. A double “equals to” sign is a comparison operator that’s used to compare if expressions are equal.
So, in the second line, where the “if” Python keyword is used, there should be a double “equals to” sign instead of a single one, since we’re not assigning values, but verifying them.
Finally, an important part of syntax is keywords (i.e., “if”, “True”, “False”, etc.). These are reserved words that cannot be used as variable names. Attempting to use variable names that are reserved as keywords will create syntax errors.
Runtime Errors vs Syntax Errors
There are two broad categories of errors in Python. While we’ve written about syntax errors in Python, there’s another type that programmers run into frequently – runtime errors. The two categories are sometimes mixed up as they can seem quite similar first.
A runtime error, however, will not prevent the program from running. They only appear once the program has begun executing and are raised only when something goes awry. A classic example of a runtime error is if a division by zero is encountered. There’s nothing in the syntax that prevents doing so, but it’s an invalid mathematical operation, so Python throws a runtime error.
It should be noted that these categories are extremely broad. Within syntax errors, you’ll find other error types nested, such as an indentation error. Python relies on spaces and indentation for code formatting, so if an indented block is added improperly, you’ll get a type of syntax error.
Syntax errors in Python are often much easier to debug and resolve. All you usually need to do is add the missing parentheses or reformat the indented block and you’re done. A runtime error will require much more debugging as there are fewer indicators as to the location and exact problem.
How to Fix Syntax Errors
Syntax errors in Python are relatively easy to fix. However, you have to follow a procedural strategy to ensure you capture everything correctly. Invalid syntax will always throw out an error with some indication of where it happened, so the first step is to review the message.
Usually, you’ll want to focus more on just the single line the interpreter provided. Unless the invalid syntax error is extremely obvious (such as mismatched punctuation or a missing colon), you’ll need to review at least a single block.
Some invalid syntax occurrences will be slightly harder to fix. An indented block may behave unusually if you tinker with it, throwing out other types of errors as well. So, you need a decent understanding of how the code should behave.
You can also use built-in debugging tools like pdb to help you out. If all else fails, the rubber ducky debugging method (wherein you explain your code loudly to someone else, even an inanimate object) is a surefire way to find ways to fix your invalid syntax.
How to Prevent Python Syntax Errors
Prevention is often the best cure, so minimizing the probability of invalid syntax will reduce many headaches down the road. There’s a few key pointers if you want to avoid such errors:
- Write clean code
Follow programming best practices when writing your code. Don’t settle for something that “just works”. You’ll often make minor modifications when coding, so clarity and cleanliness will go a long way in preventing invalid syntax errors.
- Use IDE features
Most modern IDEs have various features that make coding easier, from autocompletion to potential invalid syntax detection. Make use of the advancements made in IDEs to reduce your error rate.
- Review in pairs
Code review is an essential part of programming, but many people would like to skip it. Having someone else evaluate your code will not only help you improve but help you catch numerous errors.
- Test incrementally
Keep testing code as you write it. If something goes wrong, it’s easier to trace back 100 lines rather than 10,000.
Final Thoughts
Syntax errors are an unavoidable part of programming like grammatical errors in writing. They simply appear due to human nature, so you can’t fully eliminate them, especially if you intend to code for a long time. So, implement best practices, make use of IDEs, and don’t fret too much if you make a small mistake.
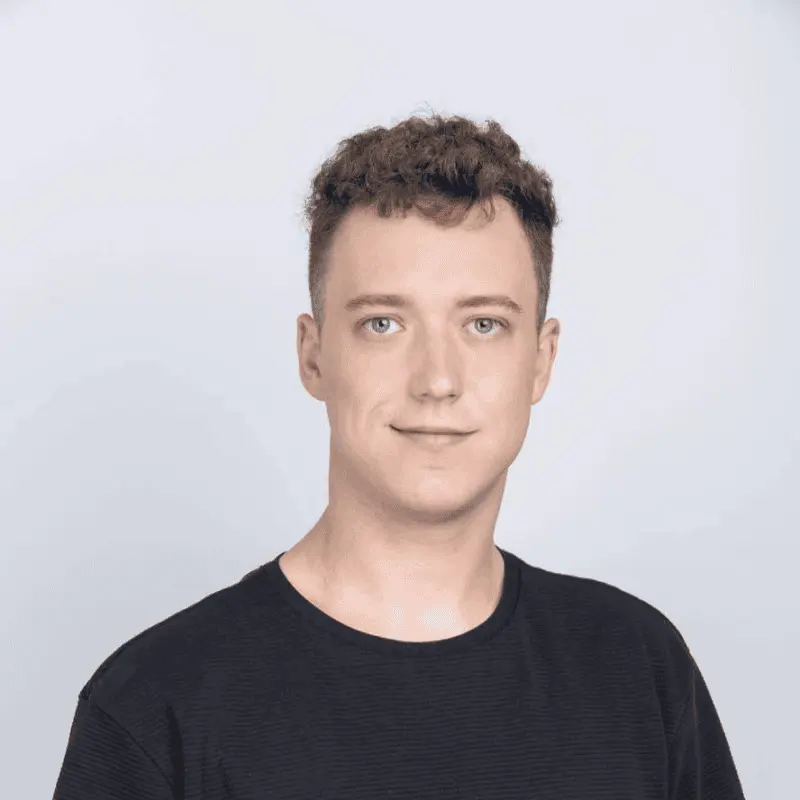
Author
Vilius Dumcius
Product Owner
With six years of programming experience, Vilius specializes in full-stack web development with PHP (Laravel), MySQL, Docker, Vue.js, and Typescript. Managing a skilled team at IPRoyal for years, he excels in overseeing diverse web projects and custom solutions. Vilius plays a critical role in managing proxy-related tasks for the company, serving as the lead programmer involved in every aspect of the business. Outside of his professional duties, Vilius channels his passion for personal and professional growth, balancing his tech expertise with a commitment to continuous improvement.
Learn More About Vilius Dumcius