How to Use Google Maps API with Python: A Comprehensive Guide
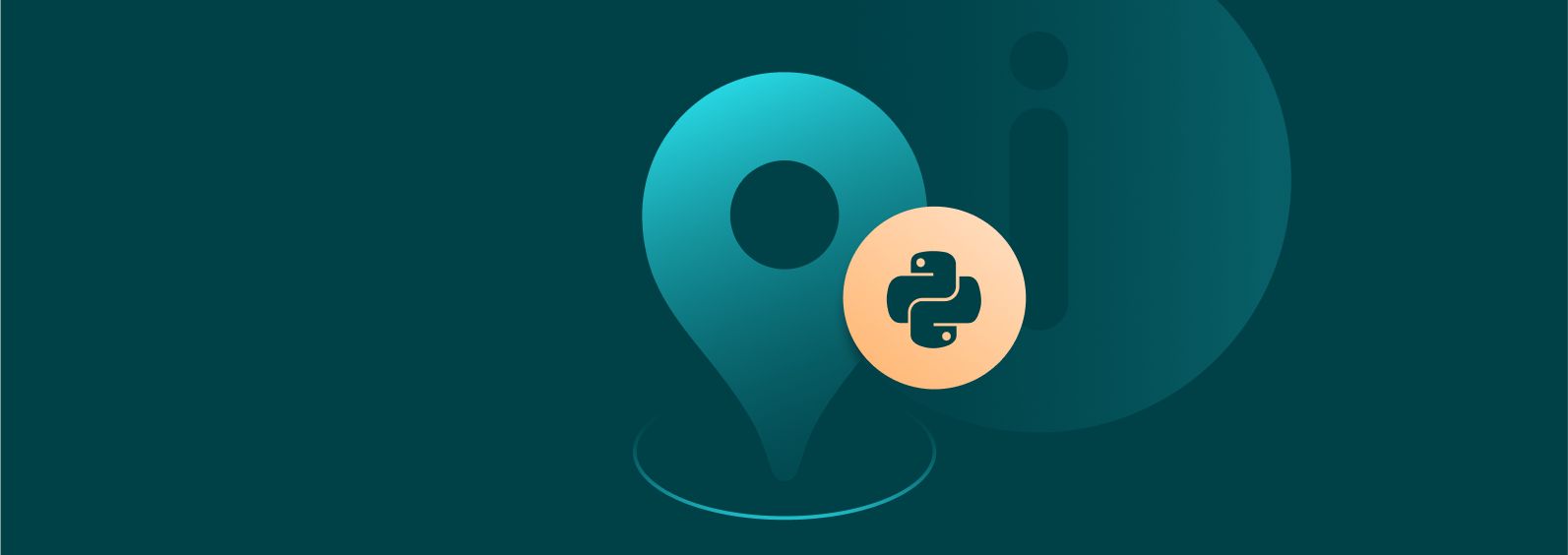
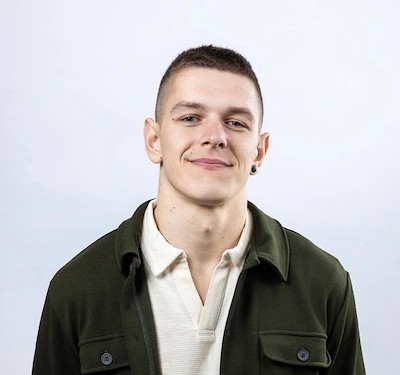
Marijus Narbutas
Key Takeaways
-
You can use the Google Maps API in Python scripts, and it benefits various use cases.
-
You can use the Google Maps API in Python scripts, and it benefits various use cases.
-
Google Maps Platform is a paid service, and you'll be billed each month, depending on your plan and the APIs used.
-
Using APIs starts by setting up the required libraries and initializing the Maps client with your API key.
-
The rest depends on the APIs you use, such as the Geocoding or Distance Matrix API.
In This Article
There’s not one but a multitude of Google APIs you can incorporate into your projects to collect and display data from Google Maps. Many popular apps and websites already use it. Chances are, you have already seen them integrated into other software.
The best part is that getting started with the Google Maps API isn’t that difficult. If your project has the budget or can fit into the free usage limits, you can make do without scraping Google Maps but using the needed APIs instead.
Can You Use Google Maps API with Python?
Google Maps Platform services, including its APIs, can definitely be integrated with Python scripts. Depending on your project and current infrastructure, you might also need JavaScript, HTML, CSS, and other languages. However, the basic functionalities of retrieving data from Google Maps can be easily accomplished with a simple Python script.
- Various web applications , such as interactive maps displaying location-based data, search, or other functionalities, use Python scripts to integrate Google Maps APIs.
- Data analysis with APIs helps to enrich datasets with geographical information, geocode them, or perform other related tasks.
- Automation tools and scripts can validate addresses, make calculations, retrieve place information, and perform other tasks with map data. Many of these use cases overlap when we compare APIs vs web scraping .
- Other projects for marketing, government, environment, Internet of Things, logistics, real estate, and tourism are also supplemented with Python scripts integrating Maps APIs.
Google provides official Python support with the google-maps-services-python library. It simplifies the process of making requests to Google Maps Platform web services, each with its own API and can be used separately. Overall, there are more than 30 APIs categorized into places, maps, routes, and environments. Here are some of them:
- Maps Embed API - allows adding interactive maps with simple HTML to web pages.
- Geocoding API - converts geographic coordinates into addresses and vice versa.
- Directions API - data on calculating routes for driving, walking, transit, etc.
- Distance matrix API - calculates travel times and distances between multiple destinations and origins.
- Places API - searching for places based on keywords and locations and retrieving their information.
- Places Autocomplete API - provides predictions for addresses and places as users type.
- Air quality API - retrieval and display of air quality data.
Besides the main Google Maps library, you might need some additional tools for specific projects, such as Google Maps API proxies and more libraries. Support and usage of libraries like requests, pandas, or others depend on your infrastructure, but in most cases, they make using Google APIs much easier.
Setting Up Your Google Maps API Key
The first thing to do before using Maps APIs is to get the API key. Getting and activating the Google Maps API doesn’t cost anything and can be done fairly easily from Google Cloud. Note that the below instructions apply as of 2025, and the interface might slightly change.
1. Access the Google Cloud console
- Open the Google Cloud Console in your web browser.
- If you haven’t already, create a Google account and log in.
2. Create a project
- If it’s your first project, you’ll see the ‘Select a project’ button on the upper left side of the screen.
- Then, click on ‘NEW PROJECT’ in the top right corner. Create a name for your project and click ‘Create’ .
- If you have multiple projects, you’ll need to choose the newly created one from the project dropdown.
3. Enable the Google Platform APIs
- In the left side menu or ‘Quick Access’ section, find the ‘APIs & Services’ tab.
- Once inside, click on the ‘+Enable APIs and Services’ button.
- A search for APIs & services will appear. Enter the name of the Maps API you need. For the code below, we’ll be using the Geocoding API and the Distance Matrix API.
- Select it and click ‘Enable’ . Note that it might take a bit for the API to be activated, especially when you enable more than one API for your key in the same project.
4. Create API credentials
- Once needed APIs are enabled, return to the ‘APIs & Services’ tab and click on ‘Credentials’ .
- Click on the ‘+Create credentials’ button at the bottom of the screen and select the ‘API Key’ option.
- A dialog box will appear with your API key. Copy it for later use in your Python scripts.
5. Restrict your API Key
- It’s highly recommended to set up restrictions on your key for security and billing purposes.
- Head back to ‘APIs & Services’ , then the ‘Credentials’ tab, and click on your newly created API Key.
- Once on the details page, you’ll find the ‘Key Restrictions’ section.
- Application restrictions refer to the type of applications (websites, servers, Android, iOS apps) that can access your API Key, increasing security.
- API restrictions specify the enabled APIs the key can call, saving you from unintended billing if something goes wrong.
6. Enable Billing
- At some point in the key creation, you will be asked to create a billing account and link it to your project.
- If you weren’t prompted to do so or skipped it, access the ‘Billing’ section on the left-hand navigation menu.
- Clicking on the ‘Link a billing account’ will guide you through the process.
How Much Does Google Maps API Cost?
Google Maps API isn’t free, and you’ll need a billing account to use it. Since March 1, 2025, Google Maps API pricing has changed and functions on a pay-as-you-go model. The pricing and free monthly calls per SKU (Stock Keeping Unit) vary depending on your plan.
- The Essentials plan gives the most common APIs with 10,000 free calls per SKU per month.
- The Pro plan provides advanced features with 5,000 free SKU calls per month.
- The Enterprise plan is made for businesses that need all the features and maximum control, with 1,000 free SKU calls per month.
Once you exceed the limit, you pay per billable event, such as a map load, geocoding request, or similar. The prices vary greatly depending on the specific API you are using.
Generally, the more popular APIs are more expensive, as not all of them have the same number of free SKU calls. Here are some of the frequently used ones to give you an idea.
- Dynamic maps cost $7 per 1,000 SKUs over the free limit.
- Geocoding API starts from $5 per 1,000 SKUs after the free limit.
- Places API is billed for $5.00 per 1,000 SKUs after the free limit.
- Routes API costs $5.00 per 1,000 SKUs after the free limit.
There are some volume discounts for power users or large corporations that scale their projects to high volumes. Other factors might also change how much you’ll be billed, so it’s best to use the pricing calculator before you start the project and track how much you’ll be charged.
Installing Python Libraries for Maps Integration
Now that you have your API key and billing account ready, you can start your Google Maps API Python project. If you haven’t already, start by installing Python from the official website and choose one of the popular integrated development environments.
Then, you need to open the command prompt or Terminal (if you are using a macOS or Linux) as an administrator and enter the following command:
pip install -U googlemaps
This will install the newest official Google Maps Python client library. Depending on your project, you might also need some other libraries. The requests library gives you more control over HTTP calls, which makes using the Google Maps API easier.
pip install requests
Another useful Python library is called pandas, and it helps with data analysis for such data as geocoding results and place or distance details.
pip install pandas
Simple Google Maps API Integration in Python
The next step is to import libraries and prepare your Google Maps API key to be used in the script. So, the beginning of your Python script should look like this:
import googlemaps
import requests
import pandas
#Replace 'API_KEY' with your actual key
gmaps = googlemaps.Client(key="API_KEY")
At this point, the script only imports the libraries and initializes the Maps client. Now it can be integrated with some other functionalities. Let's start with geocoding.
address_to_geocode = "530 5th Ave, New York, NY 10036, USA"
try:
geocode_result = gmaps.geocode(address_to_geocode)
print(f"Geocoding result for '{address_to_geocode}':")
print(geocode_result)
if geocode_result:
latitude = geocode_result[0]['geometry']['location']['lat']
longitude = geocode_result[0]['geometry']['location']['lng']
print(f"Latitude: {latitude}, Longitude: {longitude}")
else:
print("No geocoding results found for the address.")
except Exception as e:
print(f"Error occurred: {e}")
# Define helper functions
def get_latitude(geocode_result):
return geocode_result[0]['geometry']['location']['lat'] if geocode_result else None
def get_longitude(geocode_result):
return geocode_result[0]['geometry']['location']['lng'] if geocode_result else None
Now we can use the pandas library to conveniently geocode multiple addresses.
# Geocode multiple addresses
data = {'address': ["1600 Pennsylvania Avenue NW, Washington, DC 20500, United States", "530 5th Ave, New York, NY 10036, USA"]}
df = pandas.DataFrame(data)
df['geocode_result'] = df['address'].apply(lambda addr: gmaps.geocode(addr))
df['latitude'] = df['geocode_result'].apply(get_latitude)
df['longitude'] = df['geocode_result'].apply(get_longitude)
print("\nGeocoding results for multiple addresses:")
print(df)
If we use the reverse geocoding API, we can find the addresses from geographic coordinates.
latitude = df['latitude'][0]
longitude = df['longitude'][0]
reverse_result = gmaps.reverse_geocode((latitude, longitude))
print(f"\nReverse geocoding result for coordinates ({latitude}, {longitude}):")
print(reverse_result)
Further, the distance matrix API can allow us to calculate the driving distance and time between these two coordinates.
origin = (df['latitude'][0], df['longitude'][0])
destination = (df['latitude'][1], df['longitude'][1])
distance_result = gmaps.distance_matrix(origins=[origin], destinations=[destination], mode="driving")
distance_text = distance_result['rows'][0]['elements'][0]['distance']['text']
duration_text = distance_result['rows'][0]['elements'][0]['duration']['text']
print(f"\nDistance Matrix (Driving) from:\n{df['address'][0]}\nto:\n{df['address'][1]}")
print(f"Distance: {distance_text}")
print(f"Estimated Duration: {duration_text}")
Our script simplifies some parts required in real-life scenarios. It lacks error handling, and the API key should use environment variables for security. The requests library could also be used to gain more control over HTTP requests. Yet, the code should give you a start on how to use the Google Maps API.
Conclusion
These are the basics for getting started using Python for the Google Maps API. There's much more to learn using various libraries, but it depends on your use case. Be sure to check our blog for other Python applications, such as scraping Google search results .
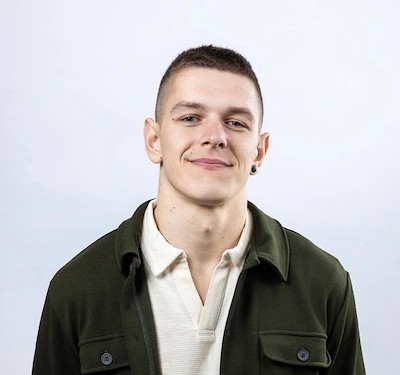
Author
Marijus Narbutas
Senior Software Engineer
With more than seven years of experience, Marijus has contributed to developing systems in various industries, including healthcare, finance, and logistics. As a backend programmer who specializes in PHP and MySQL, Marijus develops and maintains server-side applications and databases, ensuring our website works smoothly and securely, providing a seamless experience for our clients. In his free time, he enjoys gaming on his PS5 and stays active with sports like tricking, running, and weight lifting.
Learn More About Marijus Narbutas