HTTPX vs AIOHTTP vs Requests: Which to Choose?
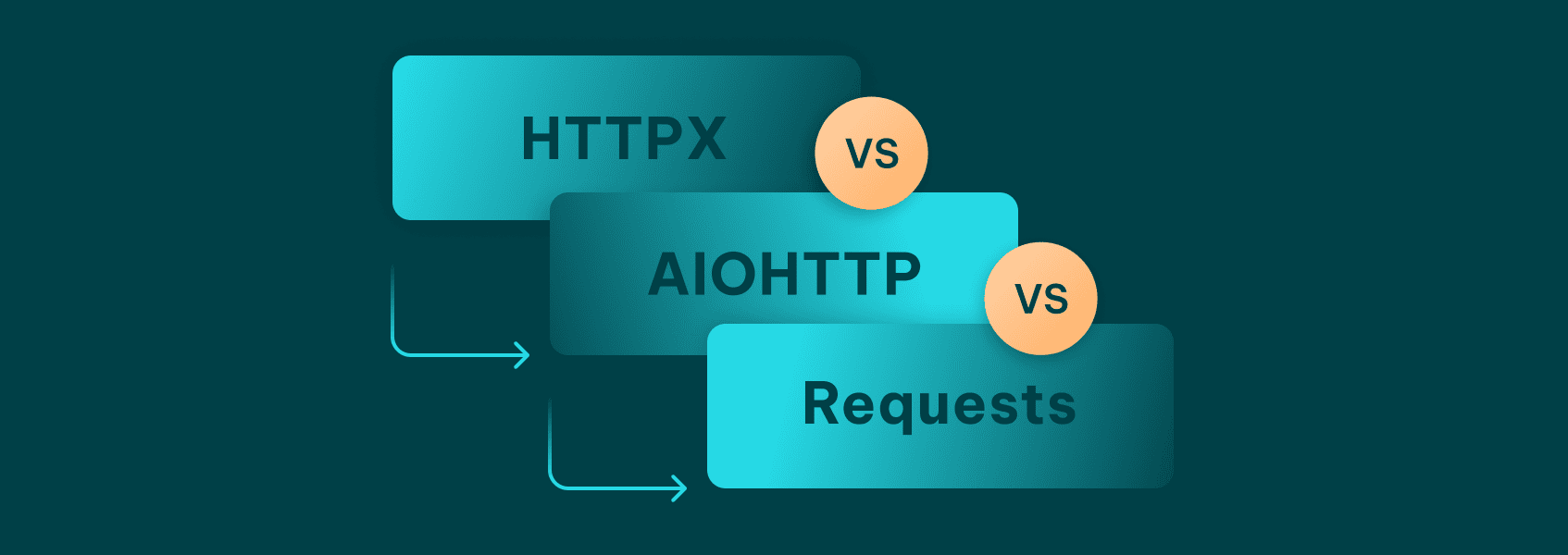
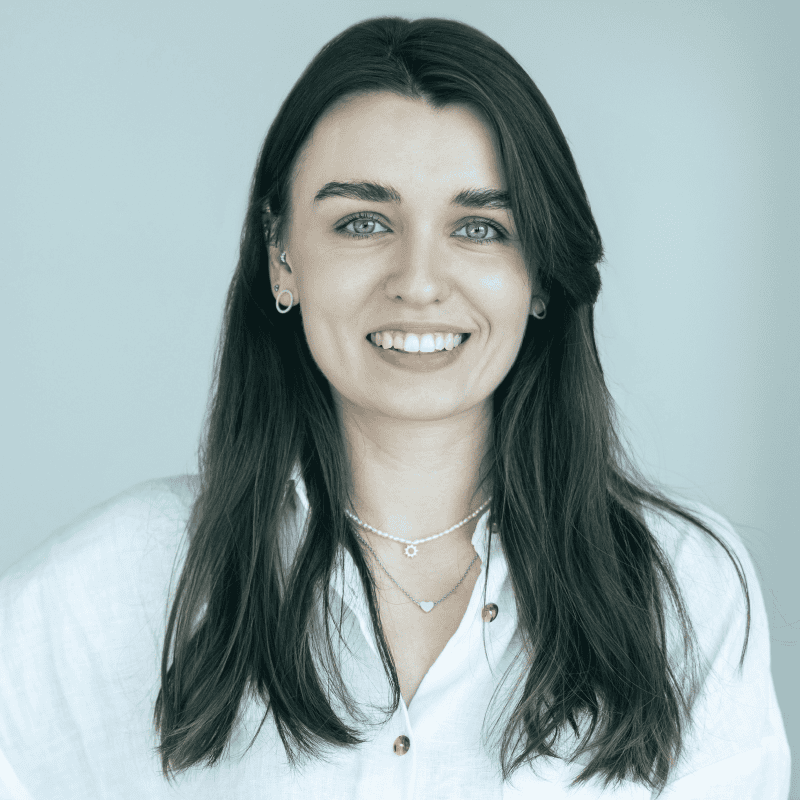
Simona Lamsodyte
Last updated -
In This Article
Python has three well-known libraries for sending HTTP (Hypertext Transfer Protocol) requests: HTTPX, AIOHTTP, and Requests. All of these have their own unique benefits and drawbacks.
Generally, you’ll want to use Requests for synchronous HTTP requests, HTTPX when you need a mix of both synchronous and asynchronous, and AIOHTTP when you need only asynchronous requests.
Requests Library
Requests is likely among the most popular Python libraries. The built-in “urllib3” and other HTTP request libraries are quite complicated and hard to parse. Requests was made to simplify the process of sending HTTP requests.
You can install the library through pip:
python -m pip install requests
Once that’s installed, you’ll need to import the library. You can send various HTTP requests by adding a method named after the request (e.g., get; post; delete).
import requests
response = requests.get("https://example.com")
print(response.text)
Simplicity is the main selling point for Requests , especially when compared to urllib3. Most of the methods and functions are extremely intuitive, making the library great for beginners.
It’s also often used in Python web development in combination with various browser automation tools such as Selenium.
Requests vs Other Libraries Rundown
Unfortunately, the library also has a few major drawbacks:
1. No async support Requests is intended for synchronous programming. While you can work around the issue, it’s often better to pick HTTPX or AIOHTTP for the job.
2. No HTTP/2 support Requests uses HTTP/1.1, which is a slower version of the protocol. When comparing Requests vs HTTPX or AIOHTTP, it’ll always be the slower library.
3. Built upon “urllib3” Requests inherits all of the benefits and drawbacks of “urllib3” since it’s built upon it. There’s less room for improvement over time, even if the library does have massive community support.
HTTPX Library
HTTPX is a library that’s inspired by Requests, but attempts to fix all of the issues associated with it. As such, HTTPX provides both async compatibility and HTTP/2 support.
These major improvements make HTTPX a popular library. Additionally, since it’s inspired by Requests, most of the methods and functions are identical.
python -m pip install httpx
Once you have the library installed, import it. Most of the methods are identical – every HTTP request is a method associated with the name of it.
import httpx
response = httpx.get("https://example.com")
print(response.text)
Async compatibility follows a fairly standard structure for sending HTTP requests.
import httpx
import asyncio
async def fetch(url):
async with httpx.AsyncClient() as client:
response = await client.get(url)
return response.status_code, response.text
async def main():
url = "https://www.example.com"
status_code, content = await fetch(url)
print(f"Status Code: {status_code}")
print(f"Content: {content[:100]}...") # Printing only the first 100 characters
if __name__ == "__main__":
asyncio.run(main())
While you can use “asyncio” with Requests, it would involve wrapping a synchronous call in an asynchronous wrapper, which might not be as efficient as using a library designed for asynchronous I/O from the ground up.
HTTPX vs Other Libraries Rundown
As with any other library, there’s some drawbacks to HTTPX:
1. Relatively new
When comparing Requests vs HTTPX, the latter is significantly newer. While it’s been getting more stable and more supported, the library still has a ways to go.
2. Higher dependencies HTTPX has more dependencies than Requests, which may be important in environments where minimizing them is crucial.
3. Learning curve HTTPX has a lot of functionality as it combines synchronous and asynchronous compatibility. Both AIOHTTP and Requests focus only on one, making them somewhat easier to learn.
AIOHTTP Library
Finally, the third member of the HTTPX vs Requests vs AIOHTTP group is a library intended for asynchronous programming only . It’s built on the “asyncio” framework, making it a library for sending HTTP requests asynchronously from the ground up.
AIOHTTP, however, is completely different from both HTTPX and Requests. It’s somewhat harder to use as sending a single HTTP request requires a few lines of code. AIOHTTP developers know that – there’s even a dedicated page to explain why .
To get started, you’ll need to first install the AIOHTTP library:
python -m pip install AIOHTTP
Setting up the entire process is a bit more complicated and requires some explanation.
import aiohttp
import asyncio
async def fetch(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
print(f"Status: {response.status}")
content = await response.text()
print(f"Body: {content[:100]}...") # Print the first 100 characters of the body
async def main():
url = "https://www.example.com"
await fetch(url)
# Run the main function
if __name__ == "__main__":
asyncio.run(main())
After importing the libraries, we’ll have to define a few functions.
The “fetch” function is the one where we’ll be sending out a GET HTTP request. Unlike regular Python functions, we have to include “async” to make it an asynchronous function.
First, we have to create a session object, which allows us to send numerous HTTP requests with the same parameters. “Async with” closes the session after the block is exited, even if an exception occurs. Part of the reason is performance and protection from leaks, the other part is syntactic sugar.
“Async with” does the same in the next line. We do, however, then use the session object to send a GET HTTP request.
Printing outputs is relatively self-explanatory. The content object, however, has one main difference – “await”, which freezes that instance of the “fetch” function. While that call is frozen, other instances of “fetch” (or other asynchronous functions in general) can be run in parallel.
As such, the power of Requests vs AIOHTTP (and, in some measure, HTTPX) is clear. With AIOHTTP you can run several HTTP requests at the same time instead of sending one after another.
AIOHTTP vs Other Libraries Rundown
As with other libraries, there are a few major drawbacks:
1. No synchronous support
It’s not a library built for synchronous requests, so when comparing Requests vs AIOHTTP, you use the former for synchronous and the latter for asynchronous. There’s no reason to do otherwise.
2. Learning curve and complexity
Out of all three, it’s the most complicated library to learn.
3. Debugging difficulties
When comparing Requests vs AIOHTTP for large code bases, the former will always be easier to debug than the latter.
Comparing HTTPX vs AIOHTTP vs Requests
When picking between all three major HTTP requests libraries, you should first decide whether you need synchronous or asynchronous compatibility. Either one will remove one of the libraries, leaving you with either Requests vs HTTPX or HTTPX vs AIOHTTP.
Additionally, consider the project scope. If you need to send a few HTTP requests for web scraping or any other means, the simpler libraries will serve you much better, leaving HTTPX vs Requests to choose from. If you need to send asynchronous HTTP requests on a major code base, there’s only one option – AIOHTTP.
Finally, optimization questions also play a part in your choice between HTTPX vs Requests vs AIOHTTP. If networking speeds and performance play a part in your project, HTTPX vs AIOHTTP are the two to choose from.
Library | Performance | Asynchronous compatibility | Ease of use | Learning curve |
---|---|---|---|---|
Requests | Slowest | No asynchronous HTTP requests | Highest | Lowest |
HTTPX | Middle-ground | Provides both types of compatibility for HTTP requests | High, comparable to Requests | Low, comparable to Requests |
AIOHTTP | Best, comparable to HTTPX | Provides only asynchronous capabilities for HTTP requests | Lowest | Highest |
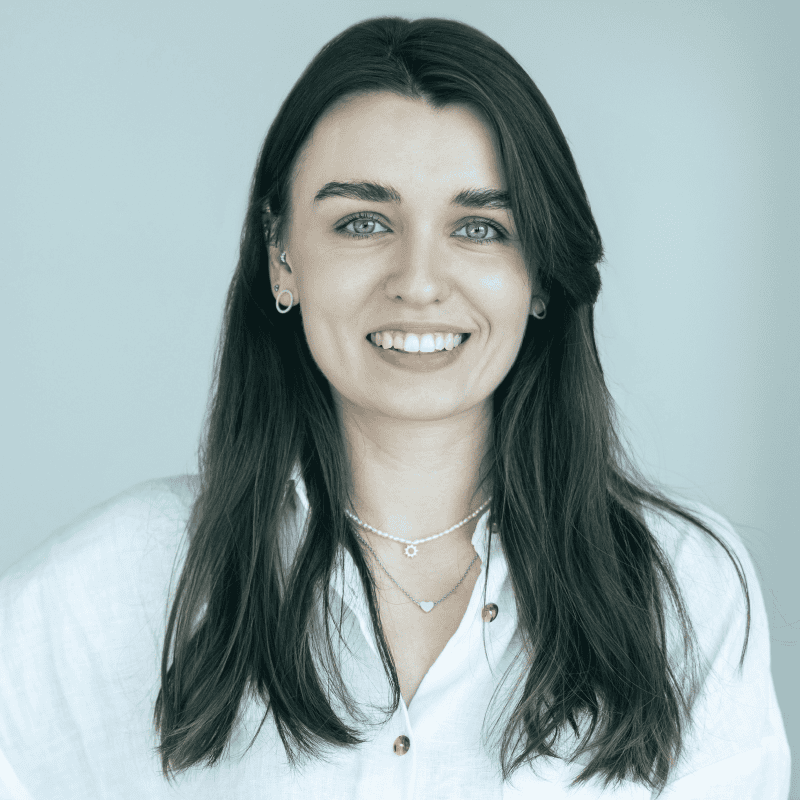
Author
Simona Lamsodyte
Content Manager
Equally known for her brutal honesty and meticulous planning, Simona has established herself as a true professional with a keen eye for detail. Her experience in project management, social media, and SEO content marketing has helped her constantly deliver outstanding results across various projects. Simona is passionate about the intricacies of technology and cybersecurity, keeping a close eye on proxy advancements and collaborating with other businesses in the industry.
Learn more about Simona Lamsodyte