How to Bypass CAPTCHA in Selenium: 5 Effective Techniques That Work in 2025
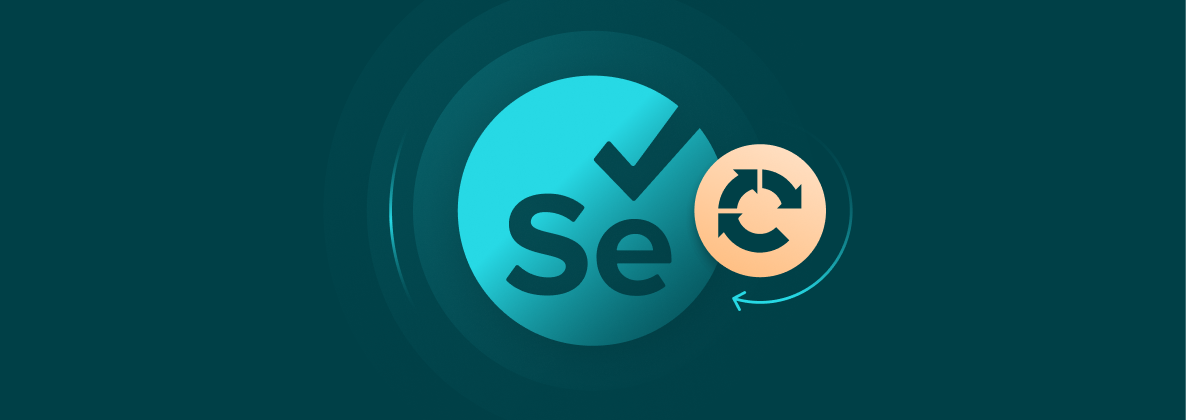
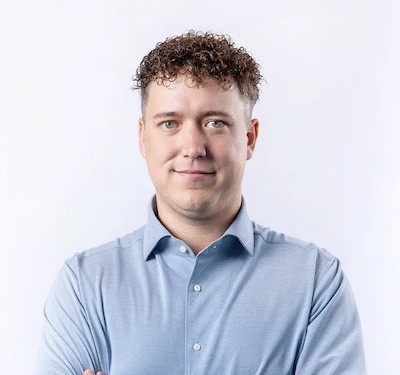
Vilius Dumcius
In This Article
CAPTCHA is a security feature meant to stop bots. It tells humans and bots apart by showing puzzles like clicking images or typing distorted text. These puzzles are meant to stop web scrapers and automated scripts from accessing a web page too fast or too often.
That’s where the trouble begins for developers who use Selenium. If you’re trying to do web scraping or automation testing, you’ll often hit a Selenium CAPTCHA bypass wall.
Before we start on how you can bypass CAPTCHA, let’s pause for a second. Some sites don’t want bots, and that’s their choice. Always follow the law and check a site’s terms of service before running a Selenium script.
1. Use Anti-CAPTCHA Services
Anti-CAPTCHA services help handle CAPTCHA in Selenium by solving them with either AI or real people. These services act like human users and send back the answer.
The logic behind this process is: you create a task specifying the CAPTCHA type, send the image or challenge to the API, and then retrieve the solution.
Python Example with Selenium
First, make sure you have the right packages installed. Then, adjust the pseudocode below with the necessary arguments with the ones of your target website:
import requests
import time
from selenium import webdriver
from selenium.webdriver.common.by import By
# Set up Selenium
driver = webdriver.Chrome()
driver.get("https://example.com")
# Locate and screenshot the CAPTCHA image
captcha_element = driver.find_element(By.ID, 'captcha_image')
captcha_element.screenshot("captcha.png")
# Read the image file
with open("captcha.png", "rb") as image_file:
image_base64 = image_file.read().encode("base64") if hasattr(image_file, "encode") else image_file.read().decode("latin1")
# Anti-Captcha API key
api_key = "YOUR_API_KEY"
# 1. Create a task
create_task_payload = {
"clientKey": api_key,
"task": {
"type": "ImageToTextTask",
"body": image_base64
}
}
create_task_response = requests.post("https://api.anti-captcha.com/createTask", json=create_task_payload).json()
task_id = create_task_response.get("taskId")
# 2. Poll for result
solution = None
if task_id:
for _ in range(20): # Max ~20s
time.sleep(5)
get_result_payload = {
"clientKey": api_key,
"taskId": task_id
}
result = requests.post("https://api.anti-captcha.com/getTaskResult", json=get_result_payload).json()
if result.get("status") == "ready":
solution = result["solution"]["text"]
break
# 3. Use the solution in the form
if solution:
driver.find_element(By.ID, "captcha_input").send_keys(solution)
else:
print("CAPTCHA solution not retrieved.")
You must still detect the CAPTCHA element correctly, which could sometimes be tricky.
Pros:
- High success rate
- Works well on image-based CAPTCHA challenges
Cons:
- Not a free option
- Delay due to response time
This method works best if you hit CAPTCHA challenges often and need quick solutions.
2. Use Undetected-ChromeDriver or Stealth Plugins
Some sites can detect if you’re using Selenium. That’s where Selenium stealth tools come in. They make the browser look like it’s being used by human users, not a bot.
It’s a custom version of ChromeDriver that avoids detection by making small changes, like hiding the Selenium import webdriver traces or adjusting window settings. Here’s an example setup:
import undetected_chromedriver as uc
options = uc.ChromeOptions()
options.add_argument("--disable-blink-features=AutomationControlled")
driver = uc.Chrome(options=options)
You’ll first need to install undetected_chromedriver, though:
pip install undetected-chromedriver
So, can Selenium bypass CAPTCHA? With Selenium stealth plugins, the site may not even show any CAPTCHAs. If using it, you should do it when CAPTCHA appears even before logging in. Or when sites block you fast due to bot activity.
It won’t always bypass CAPTCHA, but it lowers the chance of getting one.
3. Rotate Proxies and User Agents
Some sites block based on your IP or user agent string. Using a rotating Selenium proxy and different headers helps you blend in better.
Sites track patterns, and if the same IP makes a lot of requests, it signals that it could be a bot. A rotating proxy prevents that. Here’s a code snippet for proxy rotation:
from selenium import webdriver
proxy = "your.proxy.ip:port"
user_agent = "Mozilla/5.0 (Linux; Android 10; K) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/114.0.0.0 Mobile Safari/537.36"
options = webdriver.ChromeOptions()
options.add_argument(f'--proxy-server={proxy}')
options.add_argument(f'user-agent={user_agent}')
driver = webdriver.Chrome(options=options)
Make sure your Selenium import webdriver section is correct for your browser. It won’t always handle CAPTCHA in Selenium perfectly, but it keeps you from getting blocked too fast or too often.
To excel at this method, you should check out residential proxies that will help you out.
4. Leverage CAPTCHA-Solving Browser Extensions
Another way to handle CAPTCHA in Selenium is by using browser extensions. These run in the background and solve CAPTCHAs for you.
You can load an extension like Buster (for reCAPTCHA) into Chrome like this:
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
chrome_options = Options()
chrome_options.add_extension('path/to/buster.crx')
driver = webdriver.Chrome(options=chrome_options)
Note that it’s not a 100% perfect solution. Sometimes it could work, other times not that much. This method also has some limitations, such as:
- Only works for some types of CAPTCHAs.
- Can break after updates.
- Doesn’t support headless mode very well.
Still, it’s a lightweight way to bypass CAPTCHA in some simpler setups.
5. Train Your Own Solver
Now, if you’re looking for full control, you can build your own solver using OCR or machine learning. Tools like Tesseract can read text from CAPTCHA images. You’ll need to extract the CAPTCHA element, clean the image, and let OCR read it.
However, this method could be an overkill. It’s heavy. You’ll spend more time training models than solving CAPTCHAs yourself. Only use this, if:
- You’re solving the same CAPTCHA format every time.
- Other tools don’t work that well for you.
- Your target has advanced protection that makes it too difficult to bypass CAPTCHA with other methods.
- You enjoy the challenge of building new solutions.
It might work in headless mode , but you must test it first. Also, if you’re doing automation testing, this might be too much.
Conclusion
To wrap it up, there are several ways to bypass CAPTCHA with Selenium. You can use anti-CAPTCHA APIs, try Selenium stealth tools, rotate IPs and user agent strings with a rotating proxy, add browser extensions, or build your own solver.
Some of these methods support headless browsers, some won’t give the desired results in headless mode. No single trick works every time, and you’ll need to mix and match depending on the site.
If you’d like to learn more about headless browsers to tailor your approach to scraping, check out:
Also, if you’re deep into web scraping, don’t miss our guide on Selenium and Python .
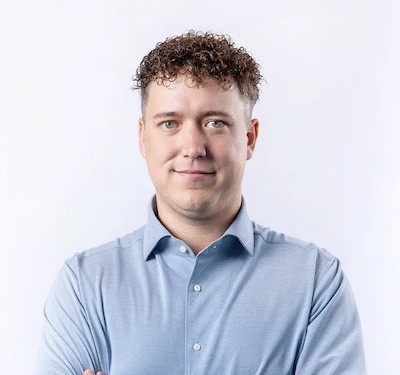
Author
Vilius Dumcius
Product Owner
With six years of programming experience, Vilius specializes in full-stack web development with PHP (Laravel), MySQL, Docker, Vue.js, and Typescript. Managing a skilled team at IPRoyal for years, he excels in overseeing diverse web projects and custom solutions. Vilius plays a critical role in managing proxy-related tasks for the company, serving as the lead programmer involved in every aspect of the business. Outside of his professional duties, Vilius channels his passion for personal and professional growth, balancing his tech expertise with a commitment to continuous improvement.
Learn More About Vilius Dumcius