API for Dummies — The Coder’s and No-coder’s Guide
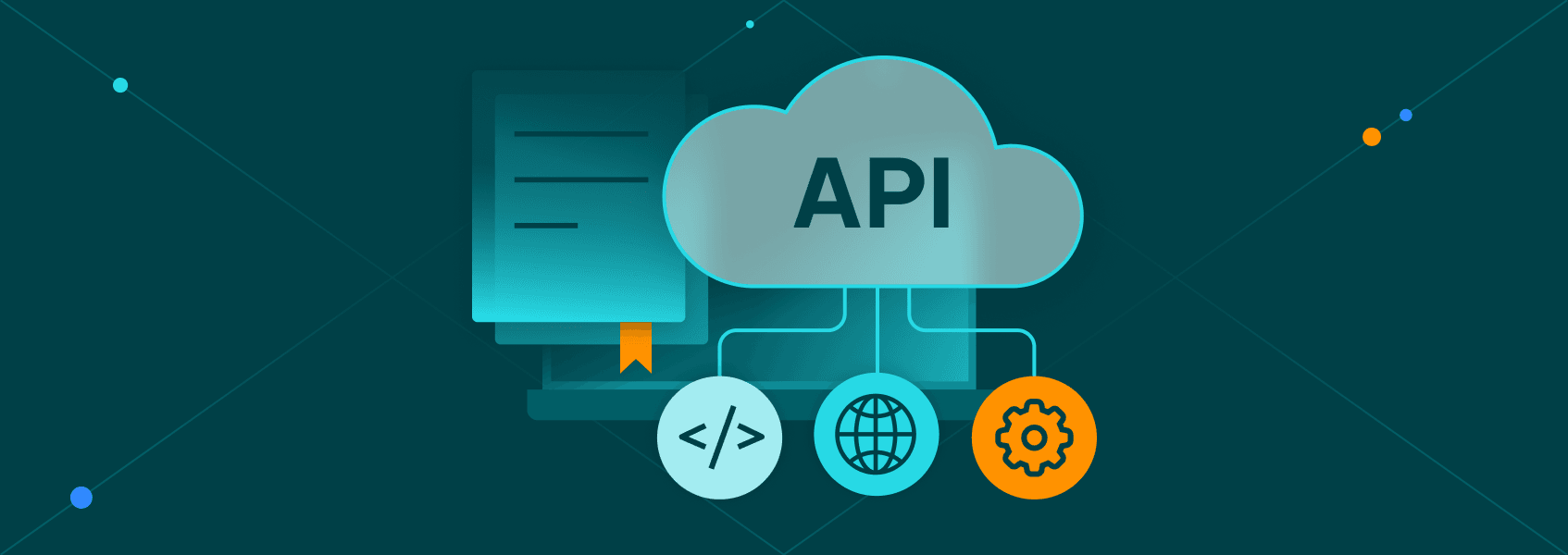
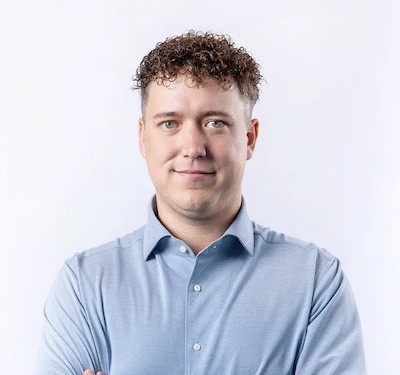
Vilius Dumcius
In This Article
This is an API for dummies guide. We will take you from the simplest uses to more complex API calls, helping coders and no-coders alike.
By the end of the day, you should be confident in your abilities to use any type of API in your projects. Also, you’ll lose any fears you might have of looking up technical docs about API endpoints.
What is an API?
API means Application Programming Interface . In essence, an API allows you to command a software using another piece of software. It contrasts with a user interface, which allows end users to interact with a piece of software.
In general, you’ll usually work with web APIs, which is a type of APIs that communicate with each other via browser requests. In this case, you send a command to a URL, the API processes it, and you read the results.
Now it gets fascinating for you as a coder (or nocoder) is that a lot of popular online services provide public APIs. This means that you can interact with their services using your own software.
For example, you can use Google’s API to allow users to login using their Gmail account. Or use GitHub’s API to create an app that manages your user’s repositories.
Companies benefit from releasing public APIs as well. They can get some money (since not all public APIs are free), but more importantly, they can get an ecosystem of apps that make their products better when they can’t create these solutions by themselves.
It’s hard for a huge company to justify building and supporting a new feature for a very specific niche case, but a developer with some free time in their hands might do it for one of their clients.
Now let’s explore how you can actually use APIs.
What Does an API Call Look Like?
From now on, whenever we say API we are referring to web APIs. They are the ones that are more relevant to your website, and we will explain other API types later.
But here is how a simple API call looks like
curl -XGET 'https://api.publicapis.org/entries?title=pet'
And this is the response that your server gets:
{
"count":2,
"entries":[
{
"API":"AdoptAPet",
"Description":"Resource to help get pets adopted",
"Auth":"apiKey",
"HTTPS":true,
"Cors":"yes",
"Link":"https://www.adoptapet.com/public/apis/pet_list.html",
"Category":"Animals"
},
{
"API":"Petfinder",
"Description":"Petfinder is dedicated to helping pets find homes, another resource to get pets adopted",
"Auth":"apiKey",
"HTTPS":true,
"Cors":"yes",
"Link":"https://www.petfinder.com/developers/",
"Category":"Animals"
}
]
The same data repeats for all entries, and there are many other entries.
You can use your browser to test this request or any other GET request without special headers.
In this request, you are loading the API using these elements:
- Method: GET
- Headers: None
- Body: none
- REST Endpoint: https://api.publicapis.org/
- API Endpoint: entries
- Parameters: title=pet
This seems like a lot of information from just one call, but let’s break down each of these components. You don’t need to memorize the names, just what each of them do.
Method
The method is the HTTP Protocol used in your request. In short, this is how your browser tells the kind of action you want to perform. There are four usual protocols for REST APIs, but you can use custom protocols if they are implemented in the API.
- GET: Read data - This is what your browser usually does
- POST: Send data - This is what your browser does when you submit a data-storage form, such as a contact form, you can specify your data using the body of the request
- PUT: Update data - You can specify your data in the body of your request
- DELETE: Delete data - You can specify which item to delete using params, the body of the request is usually ignored (or forbidden) for DELETE requests
Headers
The request headers tell more about your request and your device to the receiving server . Then can tell simple things such as the user agent/browser, to more important things, such as authentication keys.
Body
The body of a request can be used to send the actual data to add or delete on post and put requests.
REST Endpoint
The REST Endpoint is the common URL to your target API. Quite often this endpoint responds with metadata about how to use the API or what is included in it.
API Endpoint
This is the resource endpoint. In it, you tell the receiving server what kind of function you want to access. Usually, APIs provide a list of all endpoints available, along with what each of them do.
Parameters
The parameters are the arguments you are sending to the function to be executed. In our example, we are loading the /entries endpoint, which would load all entries by default. But when we specify a title, we are telling the API that we want just entries that have that string in their titles.
APIs Architectural Styles
You probably heard of REST APIs. Or maybe you recall reading something about Facebook’s GraphQL . These are architectural styles for APIs. They define how data is stored, and how you interact with the API’s resources in general terms.
It’s important to remember that no matter the style, you can use all web APIs with HTTP requests. So don’t get intimidated if an API looks very different from what you are used to. You just need to figure out what kind of request you need to make (GET, POST, PUT, DELETE), to what address, and what headers to send.
REST APIs
These are probably the easiest to see out there, because they are quite easy to build and consume. Also, they have been out there for a long time, and they were the gold standard until robust alternatives appeared (such as GraphQL).
What you need to remember about REST APIs is that they use the http method as an information about what they are doing. So you could have the same endpoint to read entries (GET /entries), to create entries (POST /entries), to update entries (PUT /entries) and to delete entries (DELETE /entries).
It accepts JSON, XML, HTML, plain text.
GraphQL
GraphQL is growing in terms of usage, and is quite a change if you are used to REST APIs only.
In it, you use the request body to specify what you want. The API defines a schema, which you can use to get, add, transform and delete data. Therefore, instead of working with multiple endpoints, quite often you have just one endpoint and you send the information you want to retrieve as the body of a POST request.
For example, the GraphQL endpoint for GitHub is this one:
https://api.github.com/graphql
And you can perform a request like this:
query {
viewer {
login
}
}
And you’ll get a result like this:
{
"data": {
"viewer": {
"login": "myGHusername"
}
}
}
You can use your request to get data, define the response structure, and even combine different fields from different resources.
Other API Architectural Styles
There are other API architectural styles such as SOAP, gRPC, RPC, Falcor, OpenAPI, Webhooks, Webhooks, MQTT, and AMQP.
They all rely on either different routes for different resources (like REST) or sending the resources you want to load along with your request (like GraphQL).
Public APIs
In general, your projects benefit from public APIs. These are APIs accessible with or without authentication. They can be free or paid, as well.
Quite frequently, free public APIs will limit the amount of data you can load. Also, they might be tracking what data you are working with, and trying to collect data about you.
If you want to work without limits, you can use IPRoyal’s residential proxies service. With it, you can use different IP addresses in each of your requests, so the API owners can’t track you down.
If you are using public APIs that require authentication, there are a few methods of doing so. It depends on the service provider, but they usually require you to send a header argument with your authentication token, or you can send it as a parameter in your request.
API Use Cases
There are many great use cases for APIs. But you can think of them as ways to perform actions on other people’s servers and data.
They are very useful for critical operations such as logins / credentials management and payment collection. If you have a small service, it can be a big burden to deal with these aspects right away.
In addition, you can use APIs to collect data from other sites. From simple social media posts to complex queries in a financial service. You can use this data or just display it to your visitors.
APIs are quite useful for automation and integration tasks. Since they remove the complexity of dealing with databases and internal functions, you can easily move around your services without much overhead. And you can use them to schedule actions, such as backups, data processing, data gathering.
How to Use APIs in No-code?
Once you have picked which APIs you want to use, it’s time to load them in your no-code app. There are quite a few ways to do it, which will depend on how your no-code builder works, and how much work you want to do yourself.
For example, if you are using Make.com (formerly integromat) you can perform an API request using the “HTTP” module, then pick the “Make a Request” action:
If you run this module, you’ll see the request results:
Then you can further manipulate the output in any way you want.
Therefore, this means that you can use almost any API even if your no-code builder doesn’t have an integration for it. As long as they have regular request modules that allow you to specify the method, headers, parameters and body, it’s all good.
What if the Request Stops Working?
It’s quite common for requests to stop working while you are testing them in your no-code builder. That’s because it is quite easy to trigger the rate limits of an API.
And if you are using free public APIs it can be worse.
They usually limit the number of requests to a specific IP address . But since all clients for your no-code builder use the same IP address, it’s likely that they have reached the rate limit a long time ago.
In this case, you’ll need to use a proxy in your requests. If your no-code builder doesn’t allow proxies, you’ll probably need to use a service to act as a bridge between your no-code builder and your API target.
Your request is going to look like this:
Be Careful With Data
When using no-code platforms, it’s quite easy to send sensitive data through it. Make sure they have the proper certifications and that your data is safe to pass through it.
Also, make sure that you don’t send your credentials to the frontend. For example, some tools allow you to save code snippets to be reused. But if you save passwords there, it’s likely that these will be visible to your end users.
How to Use APIs in Code?
One of the biggest downsides of using APIs is also one of its big advantages. You don’t control how the API performs its actions. This means that you are bound to what it does, how it does it.
Therefore, you need to handle data on your site, and be ready to dive into docs to check what kind of resources an API might have to fix what you want to fix.
On this path, one of your best friends is a good API client . You can use an app like Insomnia to check all API endpoints, and test how they work. This allows you to make sure that any issues you might be facing are related to the API itself, not a bug in your code.
Quite often, APIs have code libraries for your programming language, which makes things even easier. For example, Github has Octokit libraries and other third-party libraries.
When working with APIs, don’t forget to limit your requests. There’s nothing worse than accidentally accidentally DDoS’ing an API because you forgot to limit your requests to a reasonable amount (and this happens!).
Risks of Using APIs
APIs are great tools for your projects, but there are a few key points to consider.
First, there’s the platform risk. If you are scraping data directly, the target site can’t control what you do. But if you are using their API, they can kick you or even shut down the entire API with no prior notice.
There are data privacy risks as well. If you are sending or receiving sensitive data, you need to trust that the API is going to treat it correctly.
In addition, some APIs aren’t reliable enough. Therefore, you need to take into account the possible downtime for their services.
Conclusion
Today we looked at how you can use APIs in your projects and what you need to know about API requests. We hope you’ve enjoyed it, and see you again next time!
FAQ
What is an API in simple terms?
API is Application Programming Interface. Its counterpart is user interface. Therefore, APIs allow software to software communication, the same way an interface allows users to interact with software.
What are some API examples?
There are many great examples of companies with public APIs that you can use. There are social media APIs, sports APIs, weather APIs, recipes APIs, and much more. You can just google [sitename] API and it’s likely that the site has an API that you can use.
Is Postman a REST API?
Postman was a REST client, so it used to provide a tool for users to test API calls. Now it is an API platform since it allows you to communicate with almost any API architectural style, save code snippets, and write tests.
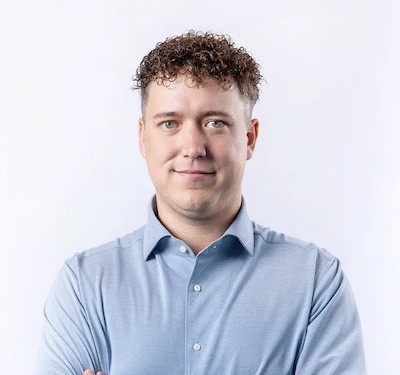
Author
Vilius Dumcius
Product Owner
With six years of programming experience, Vilius specializes in full-stack web development with PHP (Laravel), MySQL, Docker, Vue.js, and Typescript. Managing a skilled team at IPRoyal for years, he excels in overseeing diverse web projects and custom solutions. Vilius plays a critical role in managing proxy-related tasks for the company, serving as the lead programmer involved in every aspect of the business. Outside of his professional duties, Vilius channels his passion for personal and professional growth, balancing his tech expertise with a commitment to continuous improvement.
Learn More About Vilius Dumcius